


How Can I Efficiently Implement a Switch Statement for Non-Integer Values in C/C ?
Switch on Non-Integer Values in C/C
Problem:
Determining actions based on non-POD constant elements (e.g., strings) requires workarounds like nested ifs, which can be inefficient and complex. The switch statement, designed for integer values, does not directly support non-integers.
Solution 1: Macro and Template Magic (fastmatch.h)
Using macros and templates, one can create an unrolled binary search at compile time. The syntax is concise, but requires sorted case branches. This approach generates a function with implicit breaks that assigns the relevant match to a buffer.
MATCH("asd") some c++ code MATCH("bqr") ... the buffer for the match is in _buf MATCH("zzz") ... user.YOURSTUFF /*ELSE optional */ ENDMATCH(xy_match) xy_match("bqr",youruserdata);
Solution 2: C 11 Lambdas and Initializer Lists
In C 11, lambdas and initializer lists provide a cleaner solution. This approach performs a binary search on a list of key-value pairs, where the key corresponds to the non-integer value and the value is a function pointer. The found function is then invoked.
#include <utility> #include <algorithm> #include <initializer_list> template <typename KeyType, typename FunPtrType, typename Comp> void Switch(const KeyType &value, std::initializer_list<std::pair<const KeyType, FunPtrType>> sws, Comp comp) { // ... search and invoke code } Switch("ger",{ {"asdf",[]{ printf("0\n"); }}, {"bde",[]{ printf("1\n"); }}, {"ger",[]{ printf("2\n"); }} },[](const char *a,const char *b){ return strcmp(a,b)<0;});
Solution 3: Compile Time Trie (cttrie)
In C 11, a compile-time trie approach can handle unsorted case branches effortlessly. Advanced metaprogramming techniques generate a search-trie at compile time, utilizing switch statements in each trie node to efficiently redirect execution flow.
The full implementation is available at github: smilingthax/cttrie.
The above is the detailed content of How Can I Efficiently Implement a Switch Statement for Non-Integer Values in C/C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










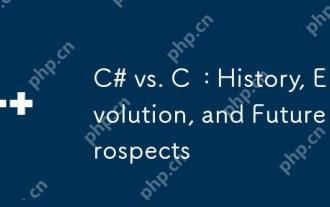
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
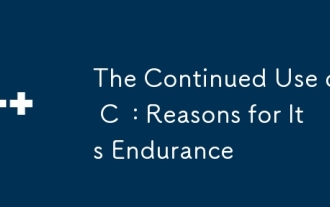
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
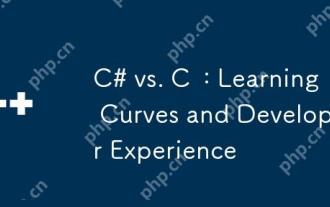
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
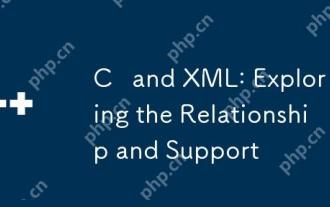
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
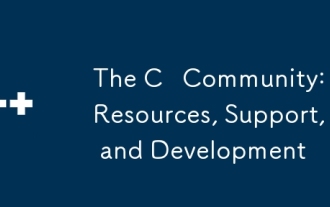
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
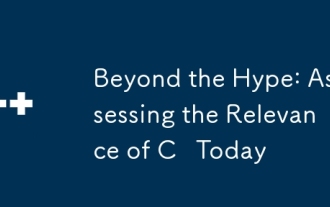
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
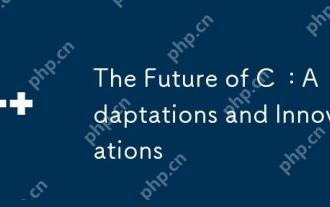
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
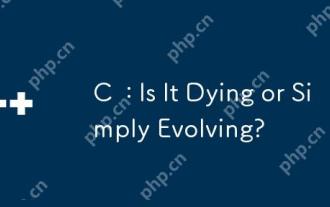
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
