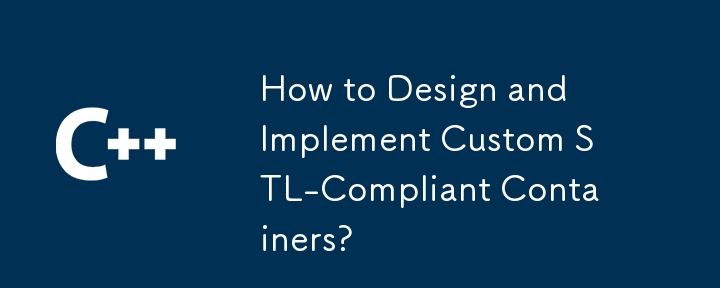
How to Create Custom STL Container
When designing new containers that behave like STL containers, several guidelines exist to ensure compatibility.
Template and Type Definitions
The container should be declared as a generic template class with appropriate type definitions:
-
typedef definitions for:
- Allocator type
- Value type
- Reference type
- Pointer type
- Difference type
- Size type
-
Class declarations for:
- Iterator type with appropriate iterator category (e.g., std::random_access_iterator_tag)
- Const iterator type
Iterators
Iterators should provide:
-
Type definitions for:
- Difference type
- Value type
- Reference type
- Pointer type
-
Constructors:
- Default constructor
- Copy constructor
- Destructor
- Assignment operator
- Comparison operators
- Increment and decrement operators (optional)
- Addition and subtraction operators (optional)
- Random access operators (e.g., operator[()]) (optional)
- Dereference operators (operator*() and operator->())
Container Class
The container class should include:
-
Constructors:
- Default constructor
- Copy constructor
- Destructor
- Assignment operator
- Comparison operators (optional)
- Begin and end iterators
- Reverse iterators (optional)
-
Element access functions:
- front() and back() (optional)
- push_front() and push_back() (optional)
- pop_front() and pop_back() (optional)
- operator[]() (optional)
-
Insertion and deletion functions:
- emplace() (optional)
- insert() (optional)
- erase() (optional)
- clear() (optional)
-
Assignment functions:
- assign() (optional)
- swap() (optional)
-
Utility functions:
- size()
- max_size()
- empty()
-
Allocator access function:
- get_allocator() (optional)
Testing
To ensure your container functions correctly, use a testing harness with a sentinel object like tester to verify its behavior. This harness can check for memory leaks and resource management issues.
The above is the detailed content of How to Design and Implement Custom STL-Compliant Containers?. For more information, please follow other related articles on the PHP Chinese website!