How Do I Convert a Go BigInt to a String or Integer?
Converting a BigInt to String or Integer in Go
In Go, a BigInt represents an arbitrarily large integer. Converting a BigInt to a string or integer is a common task when working with large numbers.
Converting to String
To convert a BigInt to a string, use the String method:
package main import ( "fmt" "math/big" ) func main() { bigint := big.NewInt(123) bigstr := bigint.String() fmt.Println(bigstr) // Output: "123" }
Converting to Integer
To convert a BigInt to an integer, use the Int64 method if you want an int64 or the Uint64 method if you want a uint64. These methods will return the integer value of the BigInt if it fits within the respective integer type. If the BigInt is too large, these methods will return 0.
package main import ( "fmt" "math/big" ) func main() { bigint := big.NewInt(123) int64Value := bigint.Int64() uint64Value := bigint.Uint64() fmt.Println(int64Value) // Output: 123 fmt.Println(uint64Value) // Output: 123 }
Note that the conversion to an integer may result in data loss if the BigInt is too large to fit into the integer type.
The above is the detailed content of How Do I Convert a Go BigInt to a String or Integer?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


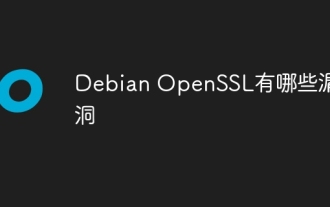
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
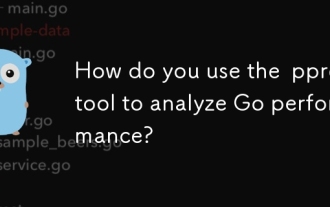
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
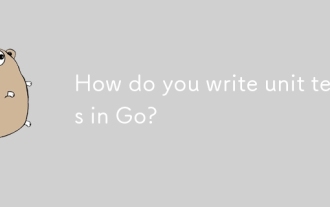
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
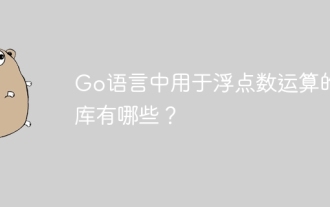
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
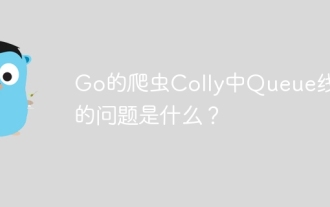
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
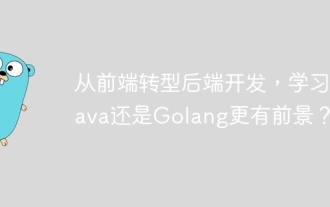
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
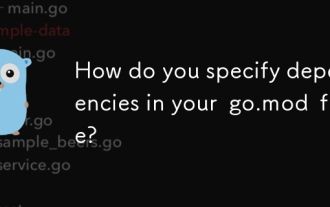
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
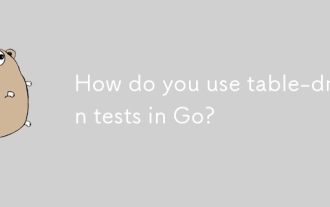
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
