Ajax: Revolutionizing Web Interaction - A Comprehensive Guide
What is Ajax?
Ajax (Asynchronous JavaScript and XML) is a set of web development techniques that enables web applications to send and retrieve data from a server asynchronously without interfering with the display and behavior of the existing page.
Core Principles of Ajax
- Asynchronous Communication: Allows web pages to update content dynamically without full page reloads
- Background Data Exchange: Enables seamless data transfer between client and server
- Enhanced User Experience: Provides responsive, interactive web interfaces
Technical Implementation Approaches
1. Traditional XMLHttpRequest
function traditionalAjax() { const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data', true); xhr.onload = function() { if (xhr.status === 200) { const data = JSON.parse(xhr.responseText); updateUI(data); } }; xhr.onerror = function() { console.error('Request failed'); }; xhr.send(); }
2. Modern Fetch API
async function modernFetch() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); updateUI(data); } catch (error) { console.error('Fetch Error:', error); } }
3. Axios Library (Enhanced Experience)
async function axiosRequest() { try { const { data } = await axios.get('https://api.example.com/data'); updateUI(data); } catch (error) { handleError(error); } }
Advanced Ajax Patterns
Debouncing Network Requests
function debounce(func, delay) { let timeoutId; return function() { const context = this; const args = arguments; clearTimeout(timeoutId); timeoutId = setTimeout(() => func.apply(context, args), delay); }; } const debouncedSearch = debounce(fetchSearchResults, 300);
Cancellable Requests
const controller = new AbortController(); const signal = controller.signal; fetch('https://api.example.com/data', { signal }) .then(response => response.json()) .then(data => updateUI(data)) .catch(err => { if (err.name === 'AbortError') { console.log('Fetch aborted'); } }); // Cancel request if needed controller.abort();
Best Practices
- Implement proper error handling
- Use loading indicators
- Minimize payload size
- Implement caching strategies
- Handle network failures gracefully
Performance Optimization
- Use browser caching
- Implement request queuing
- Minimize DOM manipulations
- Leverage HTTP/2 multiplexing
Security Considerations
- Implement CSRF protection
- Validate server-side inputs
- Use HTTPS
- Implement proper authentication tokens
Real-world Use Cases
- Social media feed updates
- Autocomplete search suggestions
- Live chat applications
- Real-time notifications
- Dynamic form validations
Emerging Trends
- WebSockets for real-time communication
- Server-Sent Events (SSE)
- GraphQL for efficient data fetching
- Progressive Web Apps (PWA)
Conclusion
Ajax remains a fundamental technique in modern web development, enabling rich, interactive user experiences.
? Connect with me on LinkedIn:
Let’s dive deeper into the world of software engineering together! I regularly share insights on JavaScript, TypeScript, Node.js, React, Next.js, data structures, algorithms, web development, and much more. Whether you're looking to enhance your skills or collaborate on exciting topics, I’d love to connect and grow with you.
Follow me: Nozibul Islam
The above is the detailed content of Ajax: Revolutionizing Web Interaction - A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










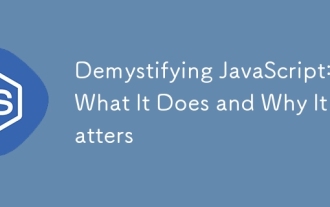
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
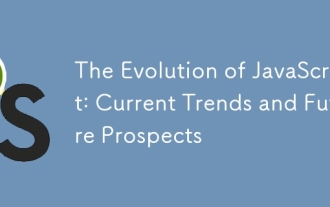
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
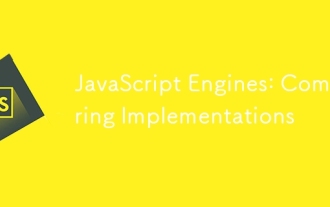
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
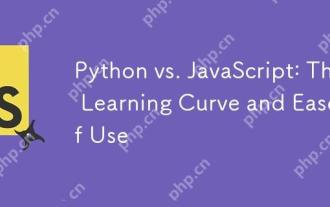
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
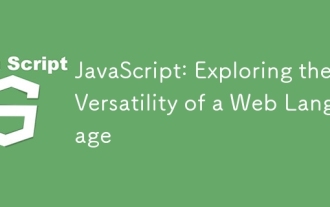
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
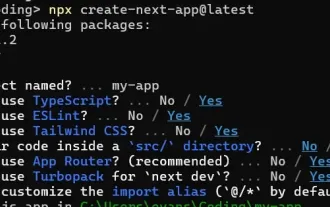
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
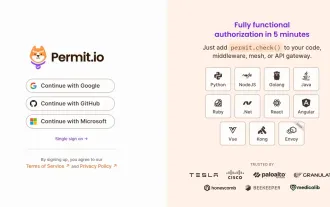
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
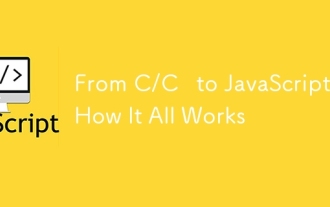
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
