


How Can I Successfully Retrieve Return Values from Python Functions Called Within a Go Program?
Calling Python Functions from Go and Retrieving Return Values
In scenarios where you need to leverage Python utilities within your Go programs, this guide will assist you in invoking Python functions and retrieving their return values.
The original code, as provided in the question:
package main import "os/exec" import "fmt" func main() { fmt.Println("here we go...") program := "python" arg0 := "-c" arg1 := fmt.Sprintf("'import pythonfile; print pythonfile.cat_strings(\"%s\", \"%s\")'", "foo", "bar") cmd := exec.Command(program, arg0, arg1) fmt.Println("command args:", cmd.Args) out, err := cmd.CombinedOutput() if err != nil { fmt.Println("Concatenation failed with error:", err.Error()) return } fmt.Println("concatentation length: ", len(out)) fmt.Println("concatenation: ", string(out)) fmt.Println("...done") }
Unfortunately, this code resulted in an empty return value. The solution lies in removing the enclosing apostrophes from the Python command:
package main import "fmt" import "os/exec" func main() { cmd := exec.Command("python", "-c", "import pythonfile; print pythonfile.cat_strings('foo', 'bar')") fmt.Println(cmd.Args) out, err := cmd.CombinedOutput() if err != nil { fmt.Println(err); } fmt.Println(string(out)) }
This alteration uncovers an interesting detail about Windows' EscapeArg function, which rewrites command line arguments by doubling backslashes before double quotes and escaping double quotes themselves. Thus, the original code was essentially escaping the Python command instead of executing it properly. By eliminating the enclosing apostrophes, the correct command is passed to Python and the function's return value is retrieved successfully.
The above is the detailed content of How Can I Successfully Retrieve Return Values from Python Functions Called Within a Go Program?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










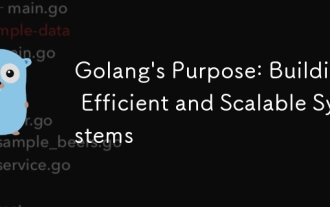
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
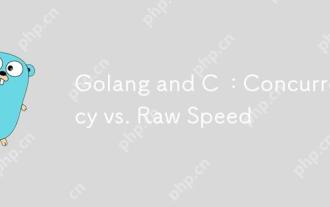
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
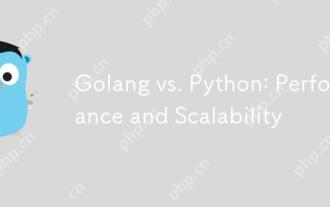
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
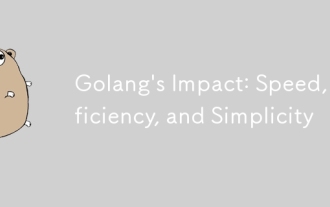
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
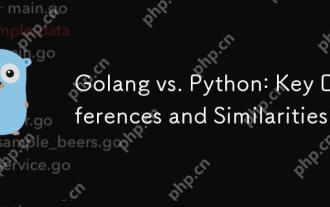
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
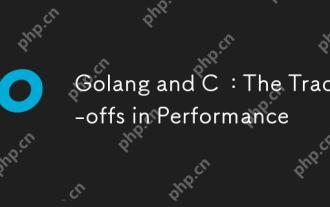
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
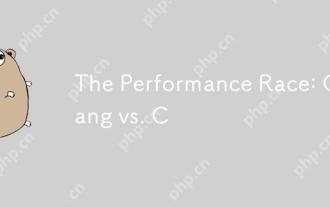
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
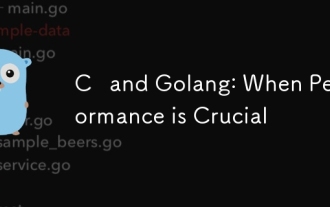
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
