


How Can I Efficiently Convert a Flat List of Key-Value Pairs into a Dictionary in Python?
Converting a Flat List to a Dictionary
In Python, converting a list of key-value pairs into a dictionary can be achieved in several ways. The simplest approach is to use the dict() function. For instance, given the list a = ['hello', 'world', '1', '2'], we can create the dictionary b using the following syntax:
b = dict(zip(a[::2], a[1::2]))
This line of code uses the zip() function to create a list of tuples, where each tuple contains a key and its corresponding value. The dict() function then converts this list of tuples into a dictionary.
Another method for converting the list to a dictionary is to use a dictionary comprehension. This approach is more concise and easier to read, especially for larger lists:
b = {a[i]: a[i+1] for i in range(0, len(a), 2)}
This comprehension iterates over the list a in pairs and assigns the first element to the key and the second element to the value.
If the list is very large, you may want to avoid creating intermediate lists. In such cases, you can use the itertools.izip() function to iterate over the list lazily, without creating a temporary list. The syntax for this approach is:
from itertools import izip i = iter(a) b = dict(izip(i, i))
In Python 3, the zip() function is already lazy, so you don't need to use izip(). You can also use the "walrus" operator (:=) to write this on one line:
b = dict(zip(i := iter(a), i))
The above is the detailed content of How Can I Efficiently Convert a Flat List of Key-Value Pairs into a Dictionary in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










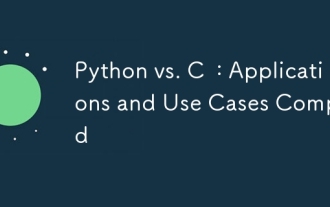
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
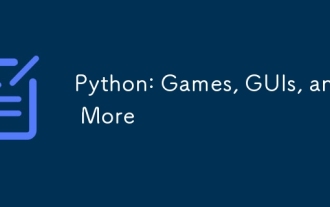
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
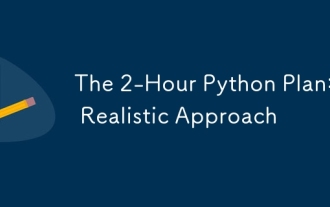
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
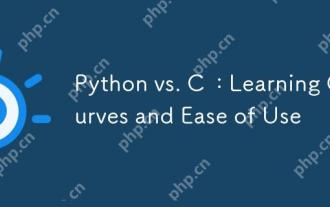
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
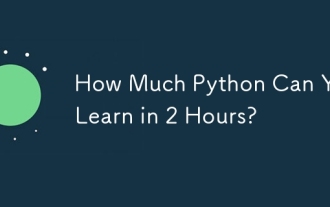
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
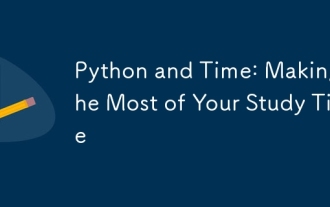
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
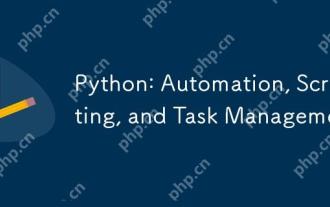
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
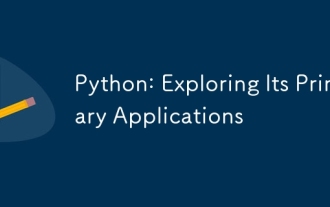
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
