


How to Generate Random Integers (and Doubles) Within a Specific Range in Java?
Math.random() Manipulation: Generating Random Integers Within a Specified Range
The Java function Math.random() generates random numbers within the range [0, 1). However, there are situations where you may need to obtain random integers within a specific range. This article demonstrates how to achieve this using Math.random().
Intention to Generate a Random Integer Between Three and Five
While the statement "(int) Math.random() * 5 3" does not correctly generate random integers within the range [3, 5], it can be corrected using a custom method:
int randomWithRange(int min, int max) { int range = (max - min) + 1; return (int) (Math.random() * range) + min; }
This method calculates the range of possible values, multiplies it by Math.random(), and adds the minimum value to ensure inclusivity.
Output Example
System.out.println(randomWithRange(3, 5)); // Output: 3
Idiot-Proofing for Incorrect Ordering
To prevent errors if min is greater than max, an updated method is:
int randomWithRange(int min, int max) { int range = Math.abs(max - min) + 1; return (int) (Math.random() * range) + (min <= max ? min : max); }
Extensions for Double Random Number Generation
A similar method can be used to generate random double-precision numbers:
double randomWithRange(double min, double max) { double range = (max - min); return (Math.random() * range) + min; }
Using this method:
System.out.println(randomWithRange(3.0, 5.0));
The above is the detailed content of How to Generate Random Integers (and Doubles) Within a Specific Range in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










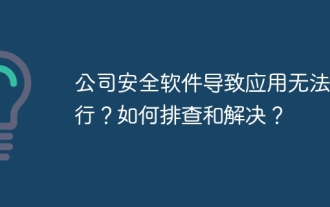
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
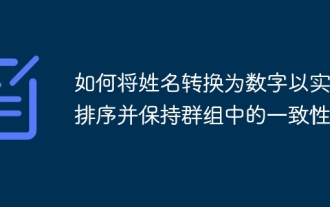
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
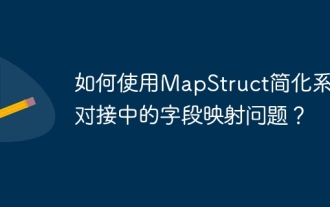
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
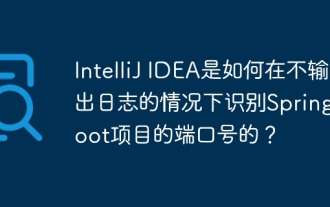
Start Spring using IntelliJIDEAUltimate version...
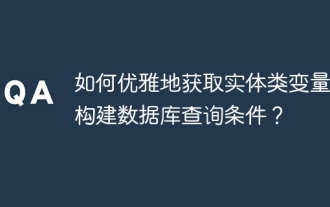
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
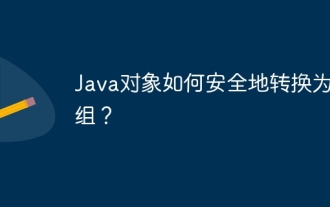
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
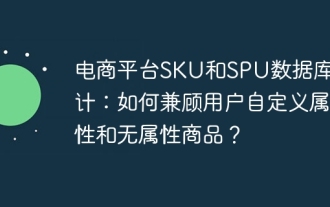
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
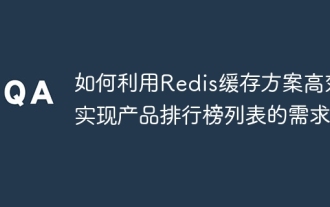
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
