How Can I Check if a String Contains Only Alphabetic Characters in Java?
Checking if a String Contains Only Letters
In programming, it is often necessary to ensure that user input adheres to specific criteria. One such requirement could be verifying that a provided string contains only alphabetic characters. This check is crucial for scenarios where numeric characters or special symbols would be considered invalid.
To address this requirement, there are two fundamental approaches: a loop-based approach for speed and a RegEx-based approach for simplicity.
Loop-Based Approach (Speed)
This approach utilizes a loop to iterate over each character in the string and check whether it is a letter. If any character fails this condition, the function returns false. This method is efficient in terms of execution speed.
public static boolean isAlpha(String name) { char[] chars = name.toCharArray(); for (char c : chars) { if (!Character.isLetter(c)) { return false; } } return true; }
RegEx-Based Approach (Simplicity)
RegEx (Regular Expressions) provide a powerful way to define complex patterns and search for them in strings. In this case, the regular expression [a-zA-Z] matches any string consisting of one or more alphabetic characters (both upper and lowercase). If the string matches this pattern, the function returns true; otherwise, it returns false.
public static boolean isAlphaRegEx(String name) { return name.matches("[a-zA-Z]+"); }
The choice of which approach to use depends on the specific requirements of the project. For situations where speed is paramount, the loop-based approach is preferable. However, if simplicity and ease of implementation are more important, the RegEx-based approach is a more elegant solution.
The above is the detailed content of How Can I Check if a String Contains Only Alphabetic Characters in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
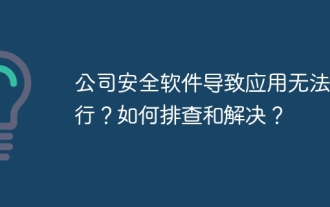
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
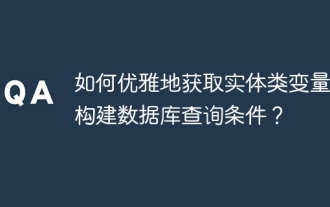
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
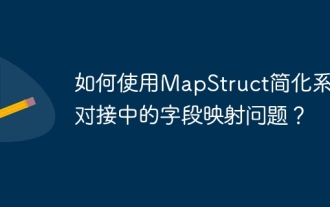
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
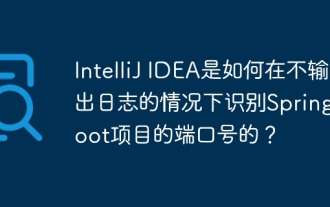
Start Spring using IntelliJIDEAUltimate version...
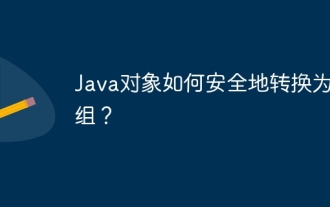
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
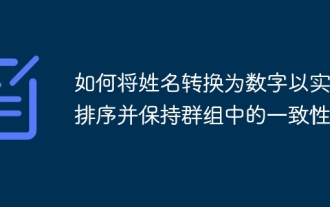
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
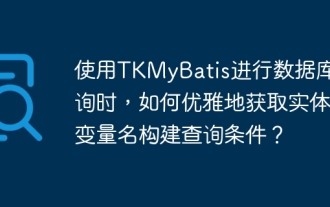
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
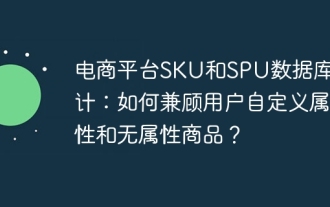
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
