Is Java Reflection Worth the Performance Hit?
Java Reflection: A Performance Trade-Off
Despite its powerful introspection capabilities, Java reflection bears a noticeable performance cost when compared to conventional object creation methods. Let's delve into the reasons behind this disparity.
Reflection vs. Constructor Invocation
Creating an object through reflection involves dynamically resolving class types, preventing the Java Virtual Machine (JVM) from performing certain optimizations. As a result, reflective operations suffer from slower performance compared to their non-reflective counterparts.
Impact on Performance
To illustrate this performance difference, consider the following code snippet:
public class Test { public static void main(String[] args) throws Exception { long startTime = System.currentTimeMillis(); for (int i = 0; i < 1000000; i++) { A a = new A(); a.doSomething(); } System.out.println(System.currentTimeMillis() - startTime); // No reflection startTime = System.currentTimeMillis(); for (int i = 0; i < 1000000; i++) { A a = (A) Class.forName("A").newInstance(); a.doSomething(); } System.out.println(System.currentTimeMillis() - startTime); // Using reflection } } class A { void doSomething() {} }
Executing this code on Sun JRE 6u10 results in significantly slower performance when using reflection:
35 // No reflection 465 // Using reflection
Optimizing Reflection
While reflection generally imposes a performance penalty, there are some strategies to mitigate its impact:
- Avoid frequent use of reflection in performance-sensitive code.
- If possible, refactor the code to avoid class lookup.
- Use cached instances of reflection metadata to minimize the number of dynamic lookups.
Conclusion
Reflection in Java provides immense flexibility but comes at a cost in terms of performance. However, with careful optimization, developers can minimize this overhead and leverage reflection's power without compromising application responsiveness.
The above is the detailed content of Is Java Reflection Worth the Performance Hit?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










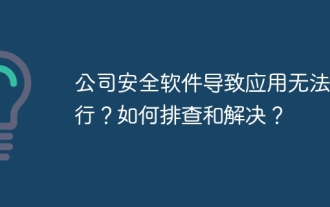
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
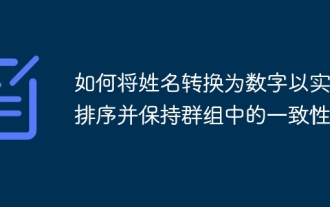
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
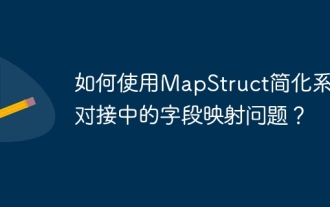
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
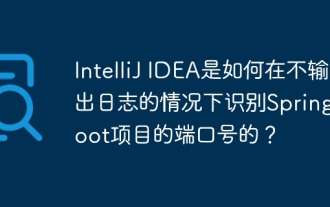
Start Spring using IntelliJIDEAUltimate version...
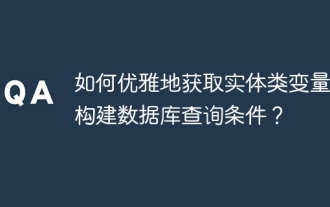
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
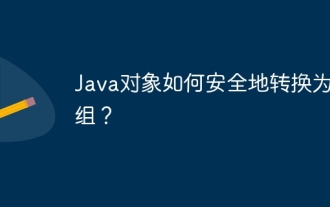
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
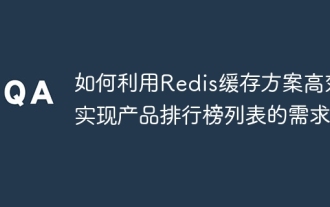
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
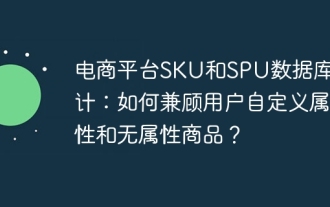
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
