


Why Does Using `sync.WaitGroup` Lead to 'fatal error: all goroutines are asleep - deadlock!', and How Can I Fix It?
Understanding and Resolving "fatal error: all goroutines are asleep - deadlock" When Using sync.WaitGroup
When attempting to coordinate the completion of multiple goroutines using sync.WaitGroup, one may encounter the error "fatal error: all goroutines are asleep - deadlock!" This cryptic message can be puzzling, especially when the code appears to be written according to the documentation examples.
The source of this error lies in the way Go handles values passed to functions. When passing a sync.WaitGroup object directly, Go creates a copy of that value, essentially passing a different object to each goroutine. This creates a disconnect between the original WaitGroup and the copies that the goroutines operate on.
The solution to this issue is to pass a pointer to the sync.WaitGroup instead. By doing so, all goroutines will reference the same underlying object and operate on its internal counters consistently. The correct code using a pointer is provided below:
import "sync" func doWork(wg *sync.WaitGroup) error { defer wg.Done() // Do some heavy lifting... request URL's or similar return nil } func main() { wg := &sync.WaitGroup{} // Use pointer to pass the WaitGroup for i := 0; i < 10; i++ { wg.Add(1) go doWork(wg) } }
With this alteration, the goroutines will properly interact with the WaitGroup object, incrementing its counter before starting their work and decrementing it upon completion. As a result, the WaitGroup.Wait() call in the main function will not block indefinitely and the program will execute as expected.
The above is the detailed content of Why Does Using `sync.WaitGroup` Lead to 'fatal error: all goroutines are asleep - deadlock!', and How Can I Fix It?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










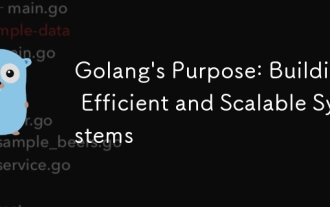
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
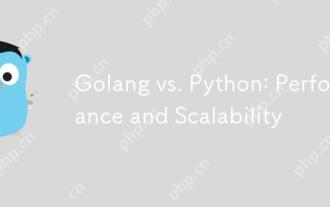
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
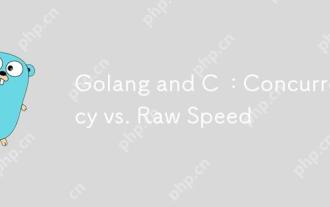
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
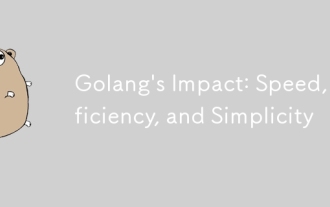
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
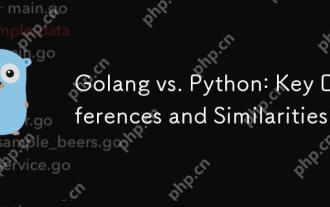
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
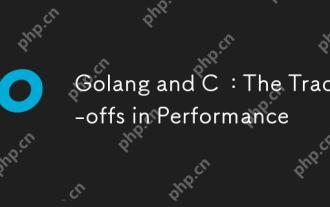
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
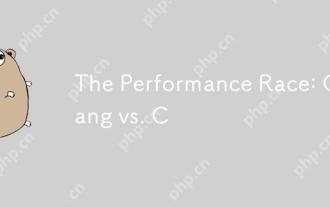
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
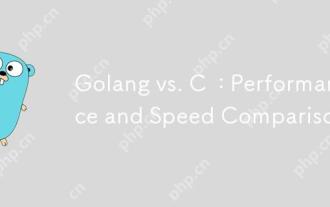
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
