


Why Do Go Slice Range Loops Create Shared Addresses When Mapping Structs?
Dec 04, 2024 pm 10:44 PMUnderstanding the Go Slice Range Phenomenon
Problem: The Mysterious Slice Range Behavior
In Go, slices are a powerful data structure that can be iterated over using the range keyword. However, in a peculiar phenomenon, when iterating over a slice of structs using a for-range, the elements in the resulting map share the same address. This behavior can be confusing, especially since the elements in the original slice should have unique addresses.
Explanation: The Gotcha of Local Variables
The key to understanding this phenomenon lies in the way variables are stored in memory. When accessing an element of the slice within the for-range loop (stu in this case), the local variable stu is holding a copy of the struct. Assigning the pointer to the local variable effectively points all the elements in the map to the same copy of the struct in memory.
Resolving the Issue: Passing Slice Element Addresses
To resolve this issue and assign the addresses of the slice elements, the code must be modified to take the address of the slice element itself. By using s[i] instead of stu, the pointer to the actual element in the slice is assigned to the map.
Example: Demonstrating the Solution
package main import "fmt" type student struct { Name string Age int } func main() { m := make(map[string]*student) s := []student{ {Name: "Allen", Age: 24}, {Name: "Tom", Age: 23}, } for i := range s { m[s[i].Name] = &s[i] // Change here } fmt.Println(m) for key, value := range m { fmt.Println(key, value) } }
Output:
map[Allen:0xc0000a6058 Tom:0xc0000a6060] Allen &{Allen 24} Tom &{Tom 23}
Conclusion
By understanding the underlying memory management behavior, we can address this slice range phenomenon in Go. By taking the address of the slice element itself, we ensure that each element in the map points to a unique struct in memory, maintaining data integrity.
The above is the detailed content of Why Do Go Slice Range Loops Create Shared Addresses When Mapping Structs?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
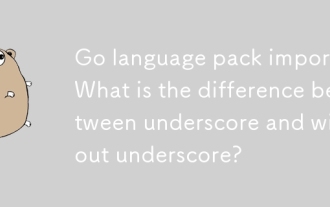
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
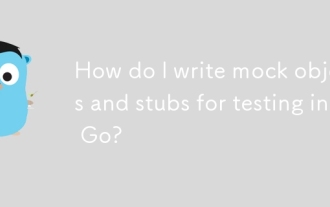
How do I write mock objects and stubs for testing in Go?
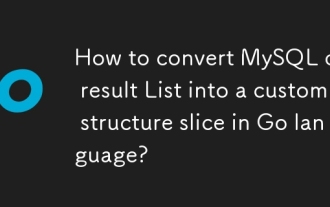
How to convert MySQL query result List into a custom structure slice in Go language?
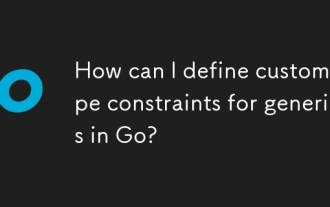
How can I define custom type constraints for generics in Go?
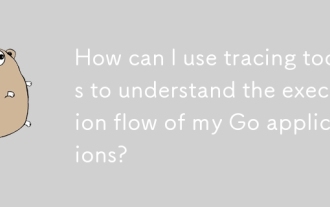
How can I use tracing tools to understand the execution flow of my Go applications?
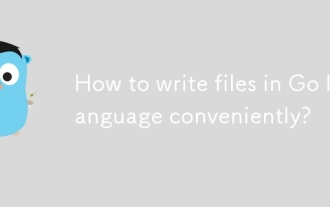
How to write files in Go language conveniently?
