


How to Disable Scrollbars While Keeping Wheel and Arrow Key Scrolling?
Disabling Scrollbars While Preserving Wheel and Arrow Scrolling Functionality
Enhancing user experience by disabling scrollbars while maintaining essential scrolling functionality can be achieved through various techniques. This article explores how to accomplish this using raw JavaScript and jQuery.
Raw JavaScript
To disable scrollbars in raw JavaScript, utilize the overflow: hidden property:
body { overflow: hidden; }
To mimic scrolling with the mouse wheel, bind the wheel event to a function that adjusts the scrollTop value:
document.body.addEventListener("wheel", function (e) { document.body.scrollTop += e.deltaY; });
For arrow key navigation, bind the keydown event to detect key presses and adjust scrollLeft and scrollTop accordingly:
document.body.addEventListener("keydown", function (e) { switch (e.keyCode) { case 37: // Left arrow document.body.scrollLeft -= 10; break; // Implement other arrow key cases... } });
jQuery
jQuery provides simplified implementations for these concepts:
// Disable scrollbars $("body").css("overflow", "hidden"); // Mouse wheel scrolling $("#example").bind("mousewheel", function (ev, delta) { $(this).scrollTop($(this).scrollTop() - Math.round(delta)); }); // Arrow key scrolling $("body").keydown(function (e) { switch (e.keyCode) { case 37: // Left arrow $(this).scrollLeft($(this).scrollLeft() - 10); break; // Implement other arrow key cases... } });
Conclusion
By employing these techniques, developers can provide a more intuitive and visually appealing user experience by concealing scrollbars while allowing users to navigate through content seamlessly.
The above is the detailed content of How to Disable Scrollbars While Keeping Wheel and Arrow Key Scrolling?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










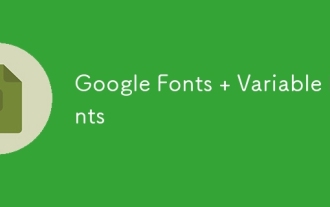
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
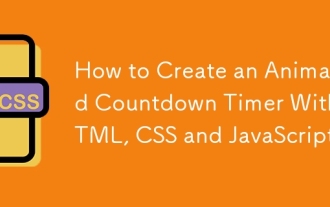
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
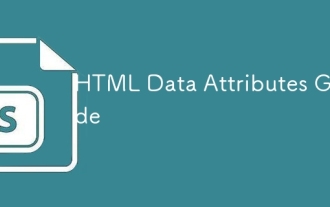
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
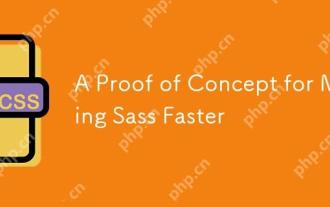
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
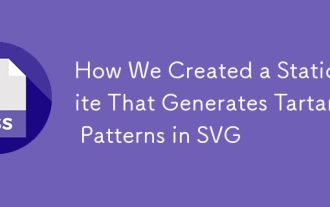
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
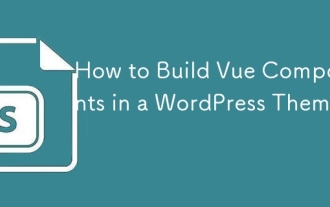
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
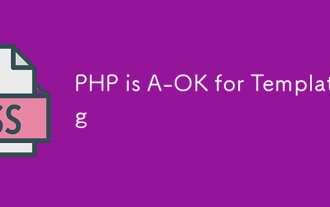
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
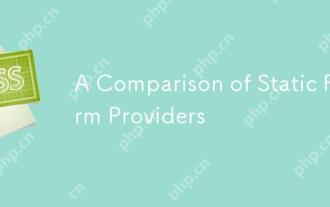
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
