


How Can We Define Common Behavior for Collections of Different Slice Types in Go?
Defining Common Behavior for a Collection of Slices
This inquiry seeks recommendations for establishing consistent behavior among collections of slices. Consider the example of working with half open intervals. Suppose you have two types of such intervals:
- ClockInterval: Represents an interval within a single day, such as "from 1:00 pm afterwards."
- Period: Represents an interval with no defined end, such as "from 9 of July of 1810 we declare independence."
Both ClockInterval and Period slices are frequently encountered in code. The challenge arises when you need to find the enclosing interval for a given time. This necessitates writing the FindEnclosingHalfOpenInterval function for both types, leading to duplicative code.
The provided code sample (https://play.golang.org/p/Cy7fFaFzYJR) demonstrates one approach, involving type conversion between slices. However, it raises questions about whether there is a more efficient way to define common behavior for multiple slice types.
Converting Slices
The appropriate method to convert a slice from one type to another is to create a new slice and iterate over the elements, converting them individually. For faster conversion, you can pre-create the resulting slice:
func ToIntervalsFromClockIntervals(clockIntervals []ClockInterval) HalfOpenIntervals { intervals := make(HalfOpenIntervals, 0, len(clockIntervals)) for _, clockInterval := range clockIntervals { intervals = append(intervals, clockInterval) } return intervals }
Composition
Alternatively, consider using composition to solve this problem. This involves creating a base struct that contains the common FindEnclosingInterval logic. For example:
type HalfOpenIntervalBase struct { intervals []HalfOpenInterval } func (base *HalfOpenIntervalBase) FindEnclosingInterval(time Time) HalfOpenInterval { // Find and return the enclosing interval using base.intervals }
You can then create separate types for ClockInterval and Period that embed this base struct:
type ClockInterval struct { HalfOpenIntervalBase } type Period struct { HalfOpenIntervalBase }
Using this approach, both ClockInterval and Period can leverage the FindEnclosingInterval functionality from the base struct, eliminating duplication.
Over-Generalization
It's important to note that over-generalization can be counterproductive. While it's tempting to pursue a universal solution that avoids code duplication, it's not always the most practical approach in Go. Duplicating code for different types is often necessary and may ultimately lead to cleaner and more maintainable code.
The above is the detailed content of How Can We Define Common Behavior for Collections of Different Slice Types in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










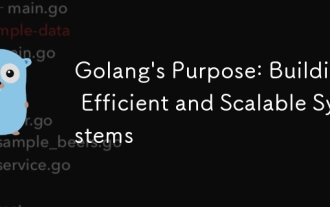
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
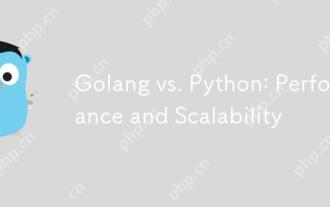
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
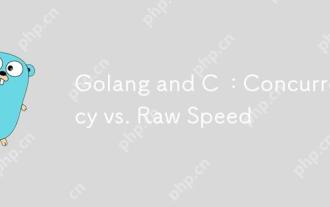
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
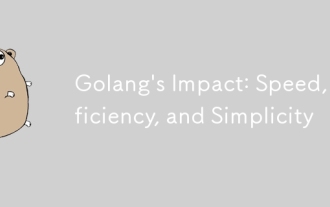
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
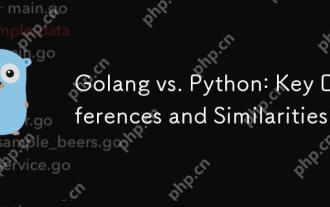
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
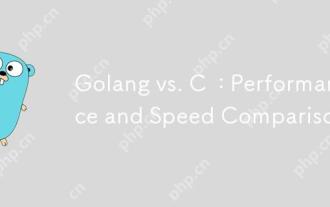
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
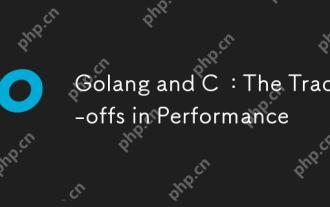
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
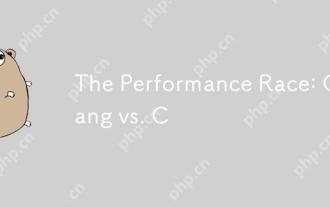
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
