


How Can I Efficiently Unmarshal XML Directly into a Go Map[string]string?
Unmarshal XML Directly Into a Map
When dealing with large XML datasets, the dual conversion process of unmarshaling into a struct and then into a map can become time-consuming. This article explores a more efficient approach to directly unmarshal XML into a map.
Problem:
The goal is to convert the following XML into a map[string]string, where the key-value pairs are extracted from child elements:
<classAccesses> <apexClass>AccountRelationUtility</apexClass> <enabled>true</enabled> </classAccesses>
To achieve this, a custom data structure that implements the xml.Unmarshaller interface is required.
Solution:
type classAccessesMap struct { m map[string]string } func (c *classAccessesMap) UnmarshalXML(d *xml.Decoder, start xml.StartElement) error { c.m = map[string]string{} key := "" val := "" for { t, _ := d.Token() switch tt := t.(type) { // TODO: parse the inner structure case xml.StartElement: fmt.Println(">", tt) case xml.EndElement: fmt.Println("<", tt) if tt.Name == start.Name { return nil } if tt.Name.Local == "enabled" { c.m[key] = val } } } }
By implementing the xml.Unmarshaller interface, the custom data structure can directly unmarshal the XML into a map[string]string. This eliminates the need for the intermediate struct conversion, resulting in a more efficient unmarshaling process.
Partial Solution and Demonstration:
A partial solution demonstrating the approach is available at https://play.golang.org/p/7aOQ5mcH6zQ. This solution includes the custom data structure and a sample XML to demonstrate the unmarshaling process.
The above is the detailed content of How Can I Efficiently Unmarshal XML Directly into a Go Map[string]string?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










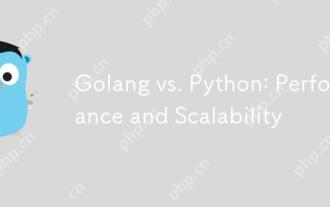
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
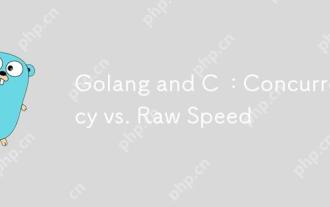
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
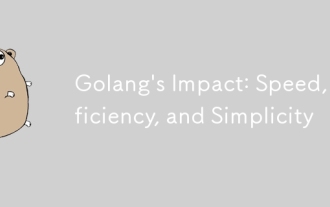
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
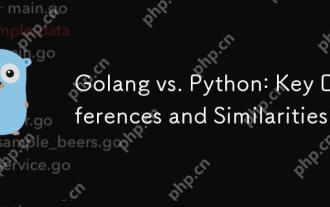
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
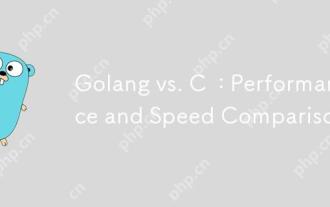
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
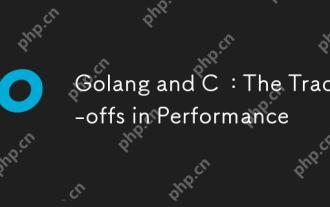
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
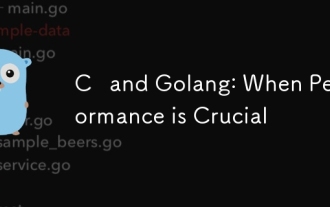
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
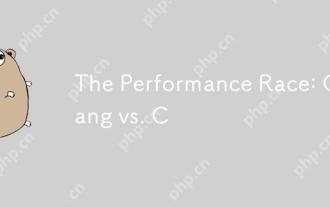
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
