How Can I Mock Struct Method Calls in Go Test Cases?
Mocking Method Calls of Structs in Go Test Cases
In Go, there is no native support for mocking method calls of structs. However, several techniques can be employed to achieve similar functionality.
One approach is to define an interface representing the relevant methods of the struct and create a mock implementation of that interface. This mock implementation can then be injected into the test case in place of the actual struct.
Consider the following code sample:
type A struct {} func (a *A) perform(url string){ // ... }
To test the invoke() function that uses this perform() method, a mock implementation of the A struct can be created:
type AMock struct { PerformFunc func(url string) } func (m *AMock) perform(url string) { if m.PerformFunc != nil { m.PerformFunc(url) } }
In the test case, the mock can be injected into the invoke() function:
func TestInvoke(t *testing.T) { mock := &AMock{} mock.PerformFunc = func(url string) { // Test logic for mock behavior } invoke(mock, "example.com") }
By setting the PerformFunc field on the mock, the behavior of the mocked method can be controlled and asserted in the test.
Another approach to mocking involves using a dependency injection framework that supports mocking. This allows for more flexible and versatile mocking capabilities, but requires additional configuration and setup.
Ultimately, the most appropriate method for mocking depends on the specific requirements and constraints of the test case and application being developed.
The above is the detailed content of How Can I Mock Struct Method Calls in Go Test Cases?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










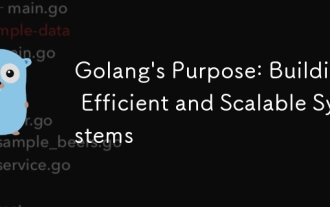
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
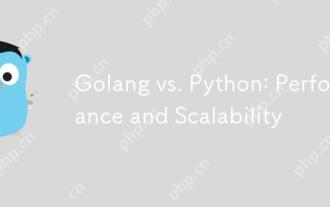
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
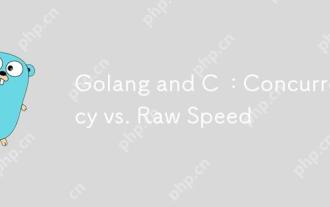
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
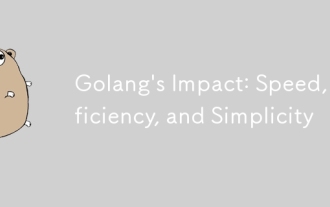
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
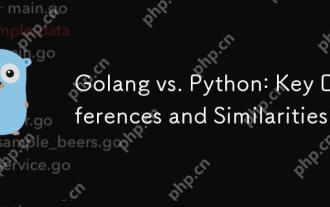
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
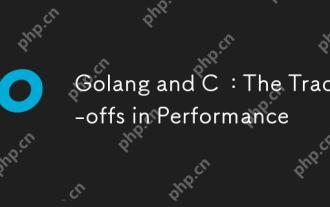
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
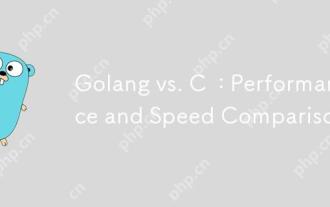
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
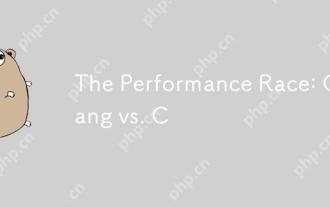
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
