Can PHP Ternary Operators Handle 'Else If' Statements?
Dec 05, 2024 am 08:55 AMA Comprehensive Guide to Incorporating 'Else If' Statements in PHP Ternary Operators
The PHP ternary operator, also known as a conditional operator, provides a succinct syntax for evaluating simple if-else statements in a single line. However, it does not natively support 'else if' conditions.
Why Ternary Operators are Unfit for 'Else If' Logic
While ternary operators offer convenience in simple scenarios, they become unwieldy when handling multiple 'else if' conditions. Nesting multiple ternaries leads to complex expressions that are difficult to read and debug.
Alternative Approaches for 'Else If' Logic
To effectively implement 'else if' logic in PHP, consider these alternative approaches:
Array Lookup Map or Dictionary
Using an associative array provides a cleaner and more maintainable solution:
$vocations = [ 1 => "Sorcerer", 2 => "Druid", 3 => "Paladin", // ... ]; echo $vocations[$result->vocation];
Nested If-Else Statements
In cases where an array lookup is not suitable, a standard nested if-else statement offers a clear and readable approach:
if ($result->vocation == 1) { echo "Sorcerer"; } elseif ($result->vocation == 2) { echo "Druid"; } elseif ($result->vocation == 3) { echo "Paladin"; } // ...
Standard Ternary Operator for Simple Conditions
For simple true/false evaluations, the standard ternary operator remains a viable option:
$value = ($condition) ? 'Truthy Value' : 'Falsey Value';
Conclusion
While the PHP ternary operator provides concise syntax for simple if-else statements, it is not well-suited for handling 'else if' logic. Alternative approaches such as array lookup maps and nested if-else statements offer more readable and maintainable solutions when handling complex conditional statements.
The above is the detailed content of Can PHP Ternary Operators Handle 'Else If' Statements?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
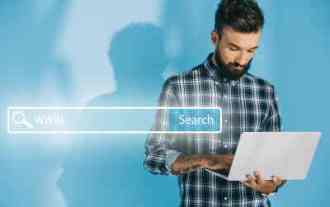
11 Best PHP URL Shortener Scripts (Free and Premium)
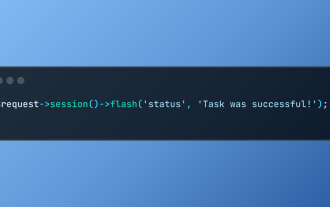
Working with Flash Session Data in Laravel
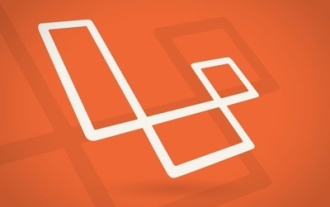
Build a React App With a Laravel Back End: Part 2, React
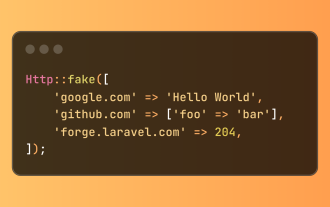
Simplified HTTP Response Mocking in Laravel Tests
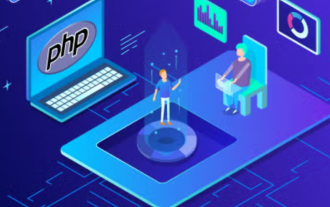
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
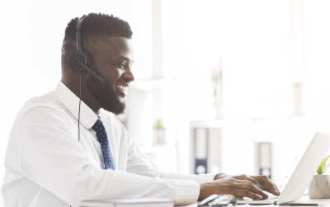
12 Best PHP Chat Scripts on CodeCanyon
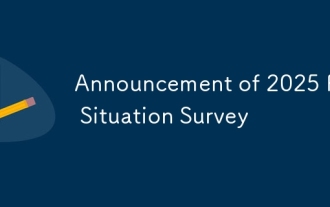
Announcement of 2025 PHP Situation Survey
