How Can I Mock Functions in Go for Effective Testing?
How to Craft Mockable Functions in Go
In Go, mocking specific functions declared within concrete types is not feasible. However, you have several options at your disposal to achieve testability:
Mocking Function Values
Function values, present as variables, struct fields, or function parameters, can be mocked in Go. Consider the following:
var Fn = func() { ... } type S struct { Fn func() } func F(Fn func())
In each of these cases, Fn is mockable.
Harnessing Interfaces
Interfaces provide an effective and preferred means of mocking in Go. Consider the following example:
type ProductRepository interface { GetProductById(DB *sql.DB, ID int) (p Product, err error) } // Real implementation type ProductStore struct{} func (ProductStore) GetProductById(DB *sql.DB, ID int) (p Product, err error) { q := "SELECT * FROM product WHERE id = ?" // ... } // Mock implementation type ProductRepositoryMock struct {} func (ProductRepositoryMock) GetProductById(DB *sql.DB, ID int) (p Product, err error) { // ... }
Code reliant upon ProductRepository can utilize ProductStore for normal execution and ProductRepositoryMock for testing purposes.
Adapting Functions through Interfaces
To retain most of your function's original structure while enabling mocking, create an interface that mirrors the methods of the type to be passed to your functions. Then, implement both a mock version of the interface and utilize it during testing.
type DBIface interface { Query(query string, args ...interface{}) (*sql.Rows, error) // ... } type DBMock struct {} func (DBMock) Query(query string, args ...interface{}) (*sql.Rows, error) { // ... } func GetProductByName(DB DBIface, name string) (p Product, err error) { ... }
The DB parameter in GetProductByName is now mockable.
The above is the detailed content of How Can I Mock Functions in Go for Effective Testing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










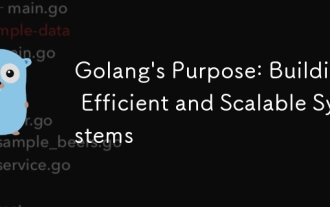
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
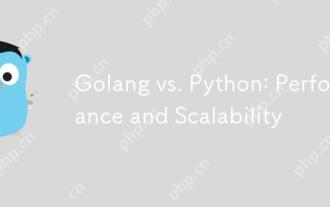
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
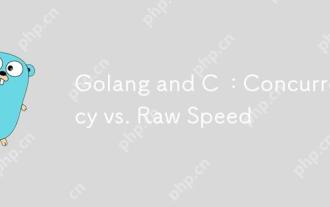
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
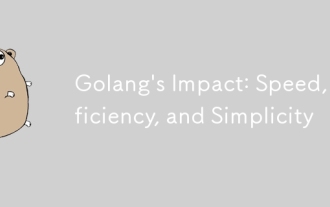
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
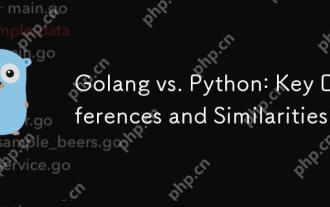
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
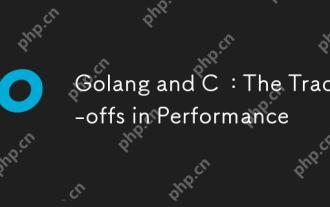
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
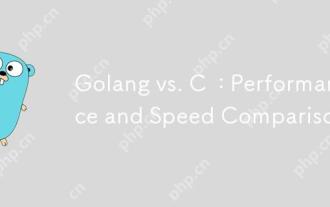
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
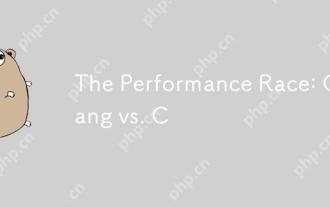
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
