Constexpr Functions or Constants: When Should I Use Which?
Constexpr Functions vs. Constants: When to Use Which
When encountering functions that consistently return the same value, such as "return 5," one may wonder why such a feature exists in C 11 and if it should replace declaring constants instead.
Constants vs. Constexpr Functions
Consider the following examples of handling a constant value:
#define MEANING_OF_LIFE 42 const int MeaningOfLife = 42; constexpr int MeaningOfLife() { return 42; }
Traditionally, one would simply declare a constant value. However, constexpr functions offer certain advantages.
When Constexpr Functions are Useful
Constexpr functions are valuable when the returned value requires more complex calculations, such as:
constexpr int MeaningOfLife(int a, int b) { return a * b; } const int meaningOfLife = MeaningOfLife(6, 7);
This allows for more readable code while still facilitating compile-time evaluation.
Compile-Time Calculations
Constexpr functions enable explicit compile-time calculation of constants, as seen in:
template<typename Type> constexpr Type max(Type a, Type b) { return a < b ? b : a; }
This allows for efficient handling of constant values.
Increased Readability
For functions like DegreesToRadians, using constexpr functions enhances readability, making it clearer that the value is being calculated at compile time:
const float oneeighty = DegreesToRadians(180.0f);
Conclusion
While declaring constants remains a valuable practice, constexpr functions offer advantages when working with more complex or readable constant values. They facilitate compile-time evaluation and enhance code maintainability. For more information on these topics, refer to http://en.cppreference.com/w/cpp/language/constexpr.
The above is the detailed content of Constexpr Functions or Constants: When Should I Use Which?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










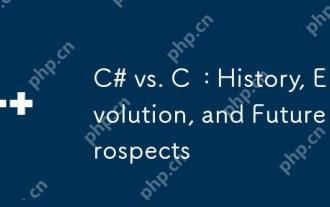
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
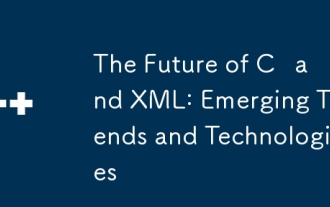
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
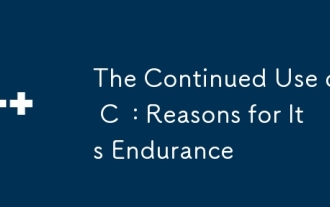
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
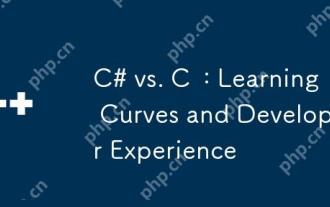
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
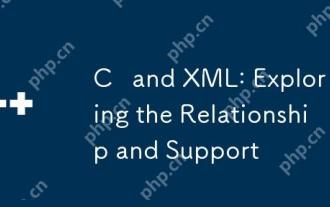
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
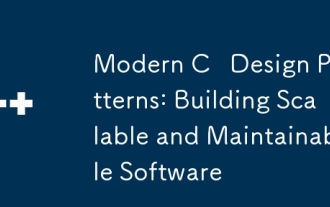
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
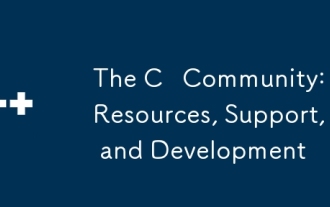
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
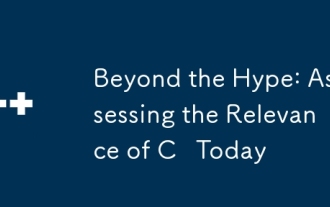
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
