How Can I Generate Random Integers Within Any Range Using Math.random()?
Math.random() Beyond Zero to One
Math.random() is a versatile method that generates random numbers between zero and one. This article explores how to harness the power of Math.random() to generate random integers within arbitrary ranges.
Generating Random Integers
While Math.random() generates floating-point numbers between 0 and 1, it's possible to use it to produce random integers within a specific range. To do this, multiply the result of Math.random() by the desired range and add the minimum value of the range.
For example, to generate an integer between zero and hundred, use the following formula:
(int) Math.floor(Math.random() * 101)
Similarly, to generate an integer between one and hundred, use:
(int) Math.ceil(Math.random() * 100)
Generating Random Integers in an Arbitrary Range
For more flexibility, it's possible to define a function that calculates random integers within a specified range. The following code achieves this:
int randomWithRange(int min, int max) { int range = (max - min) + 1; return (int)(Math.random() * range) + min; }
This function takes two arguments, min and max, representing the lower and upper bounds of the desired range. It calculates the range as the difference between max and min plus one and generates a random number within that range using Math.random(). The random number is then offset by the minimum value to produce an integer within the specified range.
For instance, the following code generates a random integer between three and five:
randomWithRange(3, 5)
Double-Precision Random Numbers
The same technique can be applied to generate double-precision random numbers within a specified range:
double randomWithRange(double min, double max) { double range = (max - min); return (Math.random() * range) + min; }
Handling Invalid Inputs
To prevent errors when the provided range is invalid (e.g., min > max), the functions can be modified to account for such situations:
int randomWithRange(int min, int max) { int range = Math.abs(max - min) + 1; return (int)(Math.random() * range) + (min <= max ? min : max); } double randomWithRange(double min, double max) { double range = Math.abs(max - min); return (Math.random() * range) + (min <= max ? min : max); }
The above is the detailed content of How Can I Generate Random Integers Within Any Range Using Math.random()?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


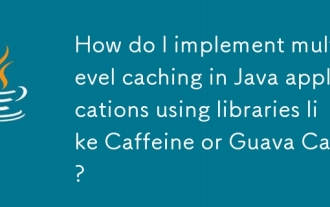
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
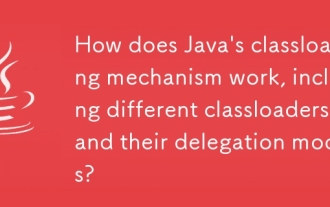
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
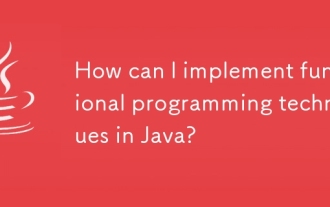
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
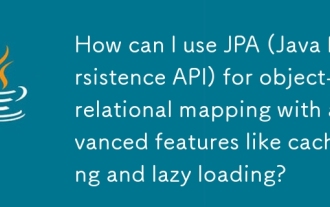
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
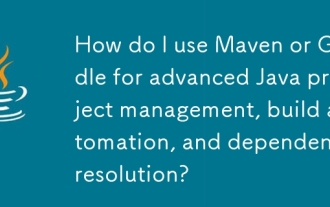
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
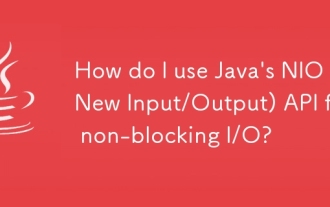
This article explains Java's NIO API for non-blocking I/O, using Selectors and Channels to handle multiple connections efficiently with a single thread. It details the process, benefits (scalability, performance), and potential pitfalls (complexity,
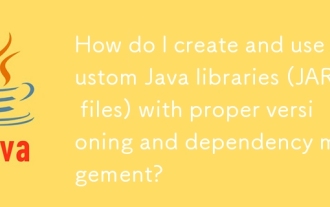
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
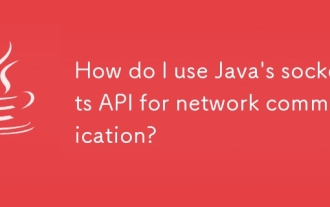
This article details Java's socket API for network communication, covering client-server setup, data handling, and crucial considerations like resource management, error handling, and security. It also explores performance optimization techniques, i
