


Java Constructors: What\'s the Difference Between Void and Non-Void Constructors?
Constructors in Java: "Void" vs. "Non-Void"
In the Java programming language, classes can have constructors, which are special methods that are called when an instance of the class is created. Constructors serve to initialize the object's state. There are two types of constructors: "void" constructors and "non-void" constructors.
"Non-Void" Constructors
A "non-void" constructor declares a return type other than void. In other words, it returns a value, usually an instance of the class itself. When a "non-void" constructor is called, a new object is created and the returned value is assigned to the reference variable that invoked the constructor.
"Void" Constructors
"Void" constructors, on the other hand, do not declare a return type. Instead, they simply execute the constructor body and initialize the object's state. They do not return a value, and therefore cannot be used to create new objects.
Constructor Visibility Issue
In Java, constructors do not have the same access modifiers as methods. A constructor's visibility must match the visibility of the class itself. For example, if a class is declared public, then its constructor must also be declared public.
Example: Constructor Visibility Impact
Consider the following two code snippets:
Example 1: Constructor with Return Type
public class Class1 { public static Integer value = 0; public Class1() { da(); } public int da() { Class1.value += 1; return 5; } public static void main(String[] args) { Class1 h = new Class1(); Class1 h2 = new Class1(); System.out.println(Class1.value); } }
Example 2: Constructor with Void Return Type
public class Class1 { public static Integer value = 0; public void Class1() { da(); } public int da() { Class1.value += 1; return 5; } public static void main(String[] args) { Class1 h = new Class1(); Class1 h2 = new Class1(); System.out.println(Class1.value); } }
Observation:
In Example 1, the output is 2. This is because the constructor calls the da() method, which increments the value field by 1.
However, in Example 2, the output is 0. This is because the constructor with the void return type does not actually call the da() method. Instead, it is a method with the same name as the class, which is distinct from the constructor.
Conclusion:
In Java, constructors are not methods. They are used to initialize an object's state and do not have return types. Attempting to declare a constructor with a return type other than void will result in a compile error.
The above is the detailed content of Java Constructors: What\'s the Difference Between Void and Non-Void Constructors?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
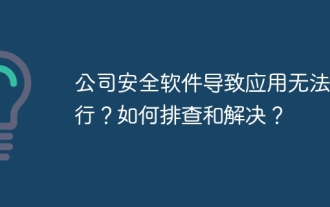
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
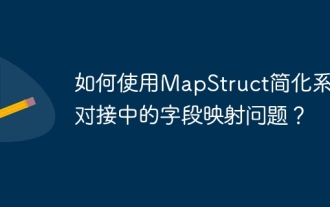
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
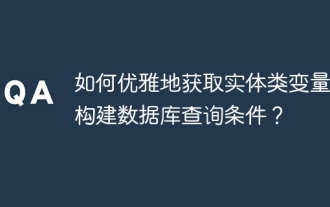
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
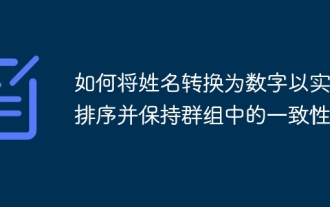
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
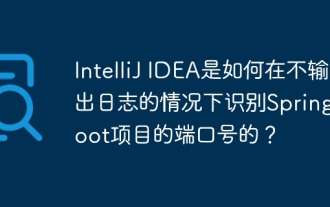
Start Spring using IntelliJIDEAUltimate version...
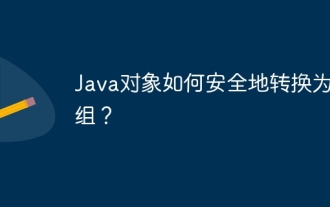
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
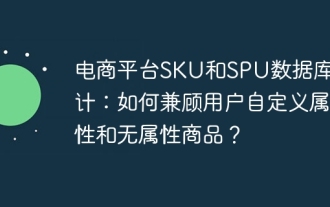
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
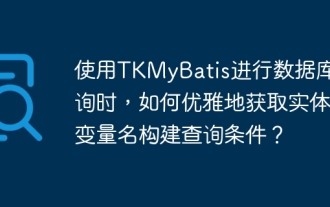
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
