How to Use Gson TypeToken with Dynamic ArrayList Item Types at Runtime?
Gson TypeToken with Dynamic ArrayList Item Type at Runtime
When working with JSON, it's common to convert JSON data into typed objects using Gson. However, you may encounter a scenario where the type of ArrayList item you need to parse is determined dynamically at runtime using reflection. In such cases, the traditional TypeToken approach may fall short.
For instance, consider this code:
Type typeOfObjectsList = new TypeToken<ArrayList<myClass>>() {}.getType(); List<myClass> objectsList = new Gson().fromJson(json, typeOfObjectsList);
This code converts a JSON string into a list of myClass objects. But what if the item type of the ArrayList should be determined dynamically?
To achieve this, you can leverage a new feature introduced in Gson version 2.8.0: TypeToken.getParameterized. This method allows you to create a TypeToken with a specified parameterized type.
Here's how you can use it:
private <T> Type setModelAndGetCorrespondingList2(Class<T> type) { Type typeOfObjectsListNew = TypeToken.getParameterized(ArrayList.class, type).getType(); return typeOfObjectsListNew; }
By passing the ArrayList class and the dynamic item type class type as arguments to TypeToken.getParameterized, you create a TypeToken that represents an ArrayList with the desired item type. This TypeToken can then be used as the parameter for Gson.fromJson to correctly parse the JSON data into the desired list type.
The above is the detailed content of How to Use Gson TypeToken with Dynamic ArrayList Item Types at Runtime?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










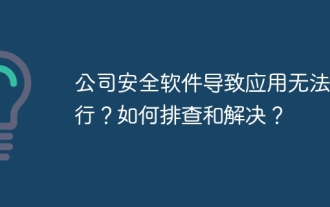
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
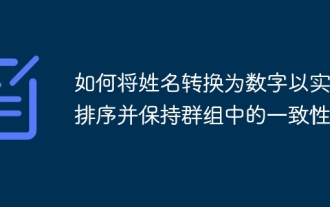
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
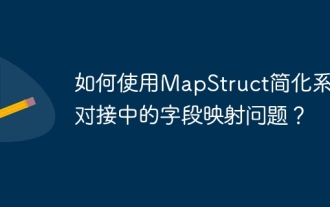
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
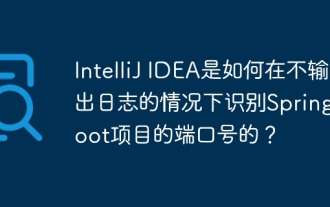
Start Spring using IntelliJIDEAUltimate version...
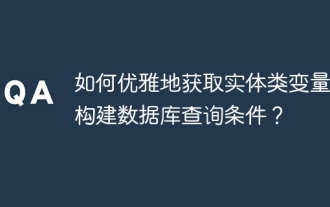
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
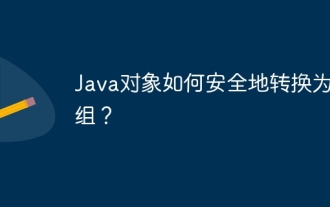
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
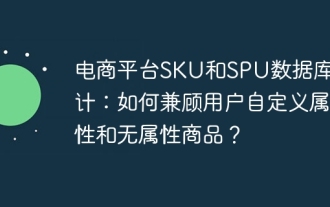
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
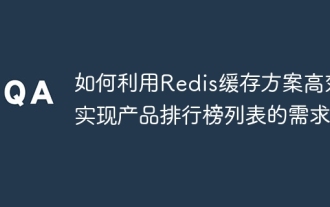
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
