How to Implement Linked Checkboxes in a JTable for Multiple Row Selection?
Selecting Multiple Rows and Checking All Checkboxes
In a JTable, where one column comprises non-editable text while the other features checkbox controls for boolean values, a common requirement is to link the checkboxes. This entails that when multiple rows are selected and any checkbox is unchecked, all other selected checkboxes also become unchecked. Similarly, if a checkbox is checked, all selected checkboxes should follow suit.
Implementing the Linked Checkboxes
Implementing a DataModel Class:
-
Extend the DefaultTableModel class to create a custom DataModel with overridden methods:
- getColumnClass(int columnIndex): Return the class type for the checkbox column (Boolean.class).
- isCellEditable(int row, int column): Allow cell editing only in the checkbox column.
Creating a Control Panel with Buttons:
- Add a control panel with buttons for clearing or checking all selected checkboxes.
Creating a SelectionAction Class:
- Implement an action class to handle button events.
- The constructor accepts a boolean value representing the desired action (clear or check).
- The actionPerformed method iterates over selected rows and updates the checkbox values accordingly.
Sample Code:**
The following code snippet demonstrates the implementation:
import java.awt.*; import java.awt.event.ActionEvent; import javax.swing.*; import javax.swing.DefaultListSelectionModel; import javax.swing.table.DefaultTableModel; public class MultipleRowSelection extends JPanel { private static final int CHECK_COL = 1; private static final Object[][] DATA = { {"One", Boolean.TRUE}, {"Two", Boolean.FALSE}, {"Three", Boolean.TRUE}, {"Four", Boolean.FALSE}, {"Five", Boolean.TRUE}, {"Six", Boolean.FALSE}, {"Seven", Boolean.TRUE}, {"Eight", Boolean.FALSE}, {"Nine", Boolean.TRUE}, {"Ten", Boolean.FALSE}}; private static final String[] COLUMNS = {"Number", "CheckBox"}; private DataModel dataModel = new DataModel(DATA, COLUMNS); private JTable table = new JTable(dataModel); private DefaultListSelectionModel selectionModel; public MultipleRowSelection() { super(new BorderLayout()); this.add(new JScrollPane(table)); this.add(new ControlPanel(), BorderLayout.SOUTH); table.setPreferredScrollableViewportSize(new Dimension(250, 175)); selectionModel = (DefaultListSelectionModel) table.getSelectionModel(); } private class DataModel extends DefaultTableModel { public DataModel(Object[][] data, Object[] columnNames) { super(data, columnNames); } @Override public Class<?> getColumnClass(int columnIndex) { if (columnIndex == CHECK_COL) { return getValueAt(0, CHECK_COL).getClass(); } return super.getColumnClass(columnIndex); } @Override public boolean isCellEditable(int row, int column) { return column == CHECK_COL; } } private class ControlPanel extends JPanel { public ControlPanel() { this.add(new JLabel("Selection:")); this.add(new JButton(new SelectionAction("Clear", false))); this.add(new JButton(new SelectionAction("Check", true))); } } private class SelectionAction extends AbstractAction { boolean value; public SelectionAction(String name, boolean value) { super(name); this.value = value; } @Override public void actionPerformed(ActionEvent e) { for (int i = 0; i < dataModel.getRowCount(); i++) { if (selectionModel.isSelectedIndex(i)) { dataModel.setValueAt(value, i, CHECK_COL); } } } } private static void createAndShowUI() { JFrame frame = new JFrame("MultipleRowSelection"); frame.add(new MultipleRowSelection()); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.pack(); frame.setLocationRelativeTo(null); frame.setVisible(true); } public static void main(String[] args) { java.awt.EventQueue.invokeLater(new Runnable() { @Override public void run() { createAndShowUI(); } }); } }
Conclusion
By implementing these classes and action listeners, it becomes possible to select multiple rows in a JTable and toggle the corresponding checkboxes collectively, enhancing the user experience and streamlining data manipulation.
The above is the detailed content of How to Implement Linked Checkboxes in a JTable for Multiple Row Selection?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
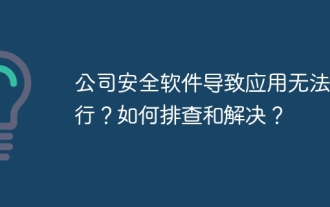
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
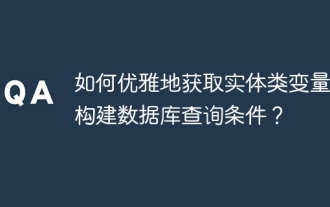
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
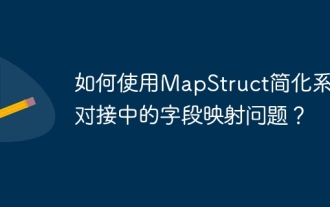
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
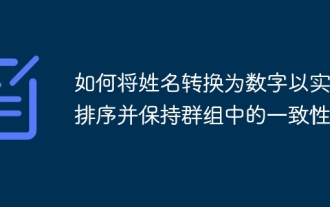
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
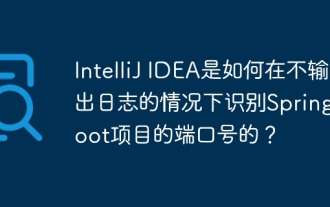
Start Spring using IntelliJIDEAUltimate version...
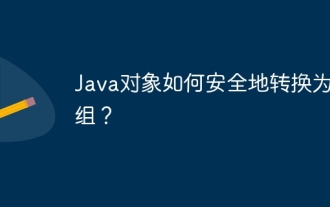
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
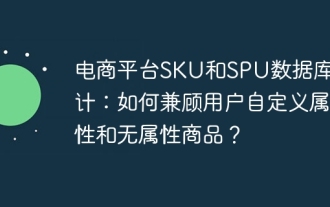
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
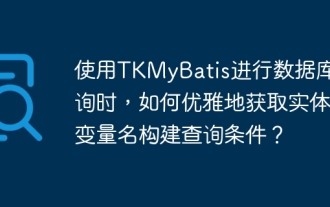
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
