


How to Prevent JDBC MySQL Connection Pool Exhaustion in Java Web Applications?
JDBC MySQL Connection Pooling: Best Practices to Avoid Exhausted Connections
When using connection pooling in Java web applications, it's crucial to avoid exhausting the pool. This can occur if connections are not closed properly, leading to the application crashing due to a lack of available connections.
Understanding the Issue
In the provided Java code, a connection pool is created using a data source and managed by a bean. However, the code fails to close connections after they are used, causing the pool to quickly run out of available connections.
Identifying and Correcting Connection Leakage
The problem arises from a lack of proper closing of connections, statements, and result sets. To resolve this, JDBC resources should be closed within a try-with-resources block:
public void create(Entity entity) throws SQLException { try ( Connection connection = dataSource.getConnection(); PreparedStatement statement = connection.prepareStatement(SQL_CREATE); ) { statement.setSomeObject(1, entity.getSomeProperty()); // ... statement.executeUpdate(); } }
For Java versions prior to 7, a try-finally block with manual resource closing should be used:
public void create(Entity entity) throws SQLException { Connection connection = null; PreparedStatement statement = null; try { connection = dataSource.getConnection(); statement = connection.prepareStatement(SQL_CREATE); statement.setSomeObject(1, entity.getSomeProperty()); // ... statement.executeUpdate(); } finally { if (statement != null) try { statement.close(); } catch (SQLException logOrIgnore) {} if (connection != null) try { connection.close(); } catch (SQLException logOrIgnore) {} } }
Closing Connections in Connection Pooling
It's essential to close connections even when using connection pooling. Connection pooling does not automatically handle closing; instead, it uses a wrapped connection that may return the connection to the pool rather than actually closing it.
Failure to close connections will prevent them from being returned to the pool and result in connection exhaustion. Proper closing ensures that connections are returned to the pool for reuse.
Additional Resources
For further information, refer to the following resources:
- [JDBC Connection Pooling in Oracle WebLogic Server](https://docs.oracle.com/cd/E24329_01/web.1211/e24485/xadatasrc.htm#CJADDHBB)
- [Closing JDBC Connections in Pool](https://www.onjava.com/pub/a/onjava/2003/05/29/closing-jdbc-connections-in-pool.html)
- [Managing JDBC Connections with Connection Pools](https://www.baeldung.com/java-jdbc-connection-pool)
The above is the detailed content of How to Prevent JDBC MySQL Connection Pool Exhaustion in Java Web Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




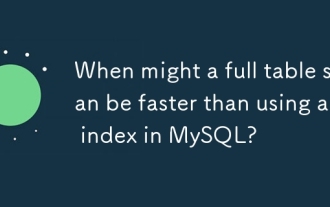
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
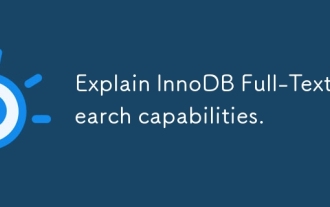
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
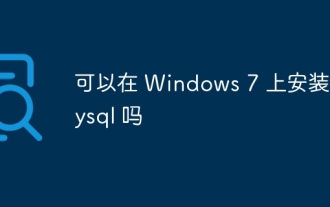
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
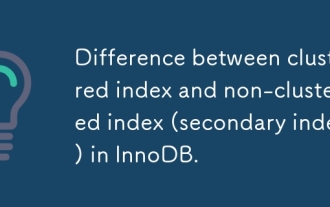
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
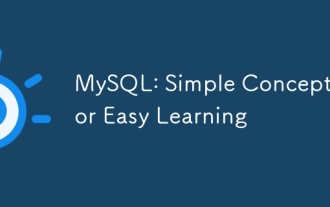
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
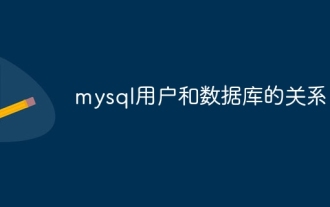
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
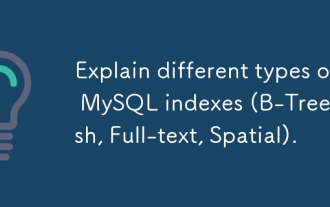
MySQL supports four index types: B-Tree, Hash, Full-text, and Spatial. 1.B-Tree index is suitable for equal value search, range query and sorting. 2. Hash index is suitable for equal value searches, but does not support range query and sorting. 3. Full-text index is used for full-text search and is suitable for processing large amounts of text data. 4. Spatial index is used for geospatial data query and is suitable for GIS applications.
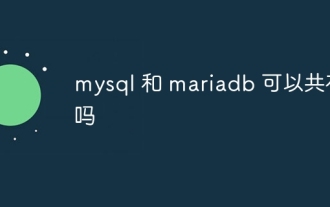
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
