How Can I Efficiently Encode a Byte Slice as an Int64 in Go?
Efficient Int64 Encoding of Byte Slices in Go
Consider the following scenario: you have an array of bytes and need to encode it as an int64 for further processing or storage. While there are various approaches to accomplish this in Go, is there a more idiomatic or efficient way to perform this encoding?
One common approach is to loop through the bytes and manually shift and merge them into the int64 variable. While this method works, it can be tedious and error-prone.
An alternative approach that is both idiomatic and efficient involves using the bitwise operators provided by Go. The following code snippet demonstrates this approach:
func main() { var mySlice = []byte{244, 244, 244, 244, 244, 244, 244, 244} data := int64(0) for _, b := range mySlice { data = (data << 8) | int64(b) } fmt.Printf("%d\n", data) }
In this example, the bitwise left shift operator (<<) is used to shift the data variable left by 8 bits in each iteration. The current byte value is then converted to an int64 and bitwise OR'd (|) with data. This process effectively appends the byte value to the left end of the data variable, creating the desired int64 representation of the byte slice.
This approach is concise, efficient, and aligns with the idiomatic style of Go. By leveraging the bitwise operators, it reduces the need for explicit loops and manual bit manipulation, making the code more readable and maintainable.
The above is the detailed content of How Can I Efficiently Encode a Byte Slice as an Int64 in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










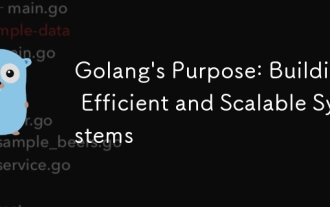
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
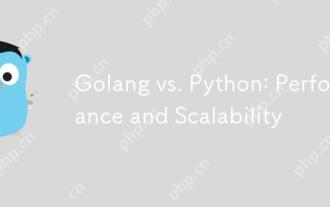
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
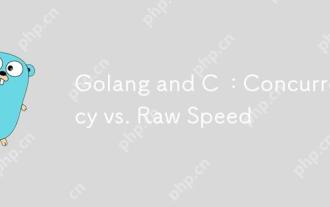
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
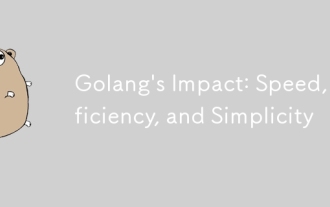
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
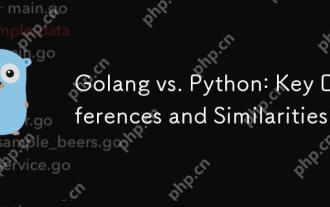
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
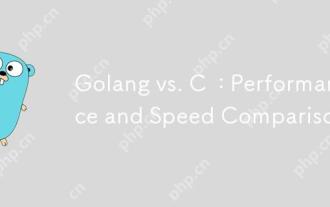
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
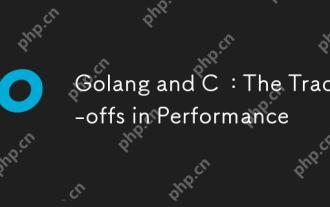
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
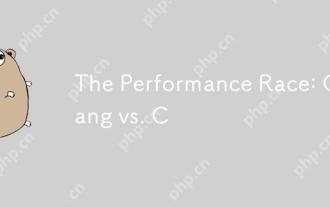
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
