


What are the differences between `echo`, `print`, `print_r`, and `var_dump` in PHP and when should I use each one?
Understanding echo, print, print_r, and var_dump in PHP
In PHP, there are multiple methods for printing or debugging variables: echo, print, print_r, and var_dump. Each of these serves a specific purpose with distinct characteristics.
echo and print: String Output
To display basic string data, you can use echo or print. These are language constructs with subtle differences. echo allows for multiple parameters and has a void return type, while print returns 1, enabling its use in expressions. Despite the slightly faster speed of echo, both are commonly employed for string output.
var_dump and print_r: Variable Debugging
For detailed debugging, var_dump provides a concise dump of variables, including their types and nested values if applicable. In contrast, print_r presents variables in a more user-friendly manner, omitting type information and presenting arrays in a structured format.
Usage Recommendations
When debugging complex data structures where type and nesting matter, var_dump offers greater insight. However, for basic troubleshooting or displaying string data, echo remains a reliable choice.
As an example, consider the following code:
$values = array(0, 0.0, false, ''); var_dump($values); print_r($values);
With print_r, the difference between integers (0 and 0.0), and boolean and empty string values (false and '') is less apparent:
array(4) { [0]=> int(0) [1]=> float(0) [2]=> bool(false) [3]=> string(0) "" } Array ( [0] => 0 [1] => 0 [2] => [3] => )
In contrast, var_dump provides a clearer distinction:
array(4) { [0]=> int(0) [1]=> double(0) [2]=> bool(false) [3]=> string(0) "" }
The above is the detailed content of What are the differences between `echo`, `print`, `print_r`, and `var_dump` in PHP and when should I use each one?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


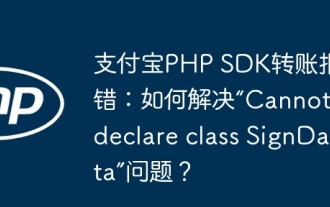
Alipay PHP...
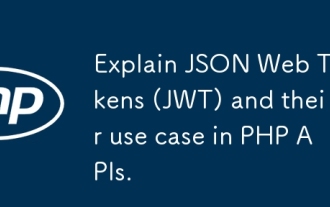
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
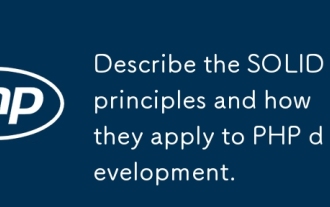
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
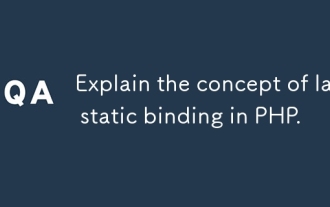
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
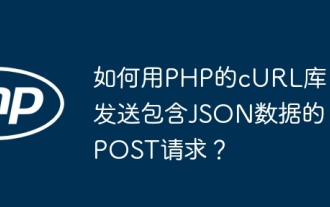
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
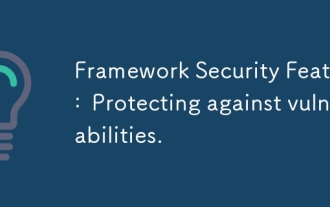
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
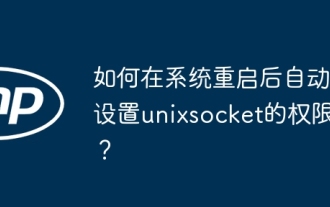
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
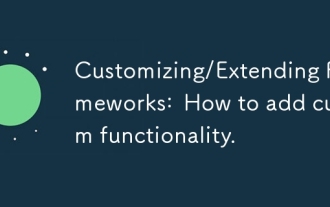
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
