


Why Do Some Decimal Numbers Appear Inaccurate When Represented as Floats in Python?
Round-Off Errors with Floating-Point Numbers in Python: Unraveling the Mystery
In the realm of numerical calculations, dealing with floating-point numbers can pose challenges due to their limited precision. While executing a Python script involving parameter variations, an unexpected issue arose: the absence of results for specific delta values (0.29 and 0.58). A closer examination revealed an underlying truth – Python's inherent inability to represent certain numbers exactly as floats.
To demonstrate this phenomenon, the following code snippet attempts to convert a range of integers to their float equivalents:
for i_delta in range(0, 101, 1): delta = float(i_delta) / 100
Intriguingly, for specific integers like 29 and 58, the resulting float values (0.28999999999999998 and 0.57999999999999996, respectively) fail to match their expected equivalents (0.29 and 0.58). This discrepancy is rooted in the fundamental limitations of floating-point arithmetic.
All floating-point systems approximate real numbers using a combination of a base, an exponent, and a fixed number of significant bits. Certain values, particularly those with fractional parts that cannot be expressed exactly as a power of two, are inherently challenging to represent accurately. Consequently, these values are rounded or approximated during storage and computation.
To visualize the impact of this rounding, a Python script was devised to demonstrate the discrepancies between the actual integers and their float approximations:
import sys n = int(sys.argv[1]) for i in range(0, n + 1): a = int(100 * (float(i) / 100)) if i != a: print i, a
While there seems to be no discernible pattern in the numbers exhibiting this behavior, the underlying principle remains constant: any number that cannot be precisely represented as a combination of exact powers of two faces the possibility of being approximated when stored as a float.
To delve deeper into the complexities of floating-point arithmetic and its consequences in computing, exploring resources like "What Every Computer Scientist Should Know About Floating-Point Arithmetic" is highly recommended. Understanding these nuances is paramount for navigating the pitfalls of numerical analysis and ensuring the accuracy of your computations.
The above is the detailed content of Why Do Some Decimal Numbers Appear Inaccurate When Represented as Floats in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










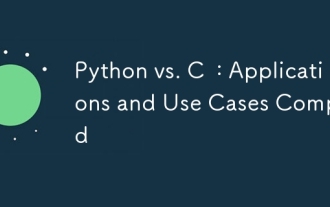
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
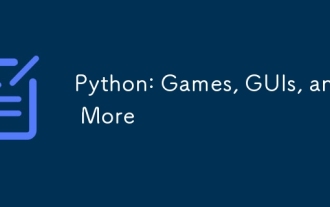
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
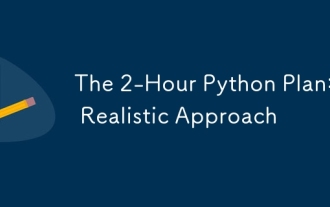
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
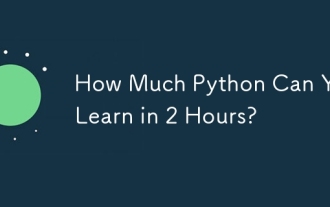
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
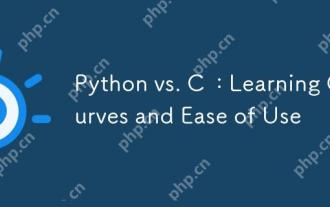
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
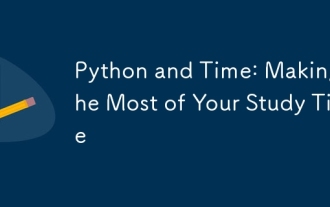
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
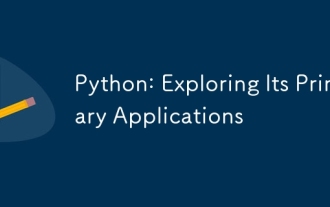
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
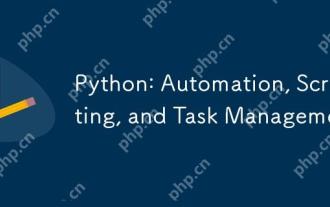
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
