


How Can I Use Custom Deleters with std::unique_ptr Members for Third-Party Classes?
Dec 06, 2024 am 03:17 AMUsing Custom Deleters with std::unique_ptr Members
In object-oriented programming, it is often desirable to utilize member objects managed by unique pointers (std::unique_ptr). However, when dealing with third-party classes that have custom memory management requirements, employing a custom deleter can prove beneficial.
Consider a scenario where you have a class (Foo) with a member managed by a unique pointer (std::unique_ptr<Bar>). The third-party class (Bar) provides create() and destroy() functions for memory management.
To address this, you can implement a custom deleter within your Foo class, using syntax similar to the following:
class Foo { private: std::unique_ptr<Bar, void(*)(Bar*)> ptr_; // ... public: Foo() : ptr_(create(), destroy) { /* ... */ } // ... };
In this example, create() and destroy() are assumed to be free functions that adhere to the following signatures:
Bar* create(); void destroy(Bar*);
By specifying destroy as the second template argument of the unique pointer, you effectively instruct the pointer to invoke destroy() when the managed object is deleted.
This approach allows you to seamlessly manage the memory of your third-party member object while maintaining the benefits of using unique pointers, such as automatic resource deallocation and ownership semantics.
The above is the detailed content of How Can I Use Custom Deleters with std::unique_ptr Members for Third-Party Classes?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
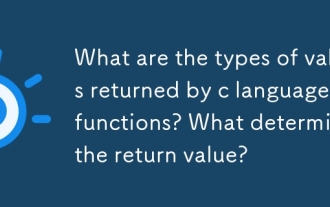
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
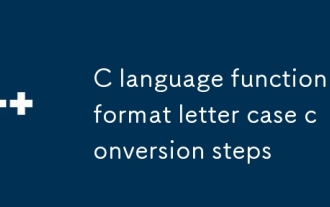
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
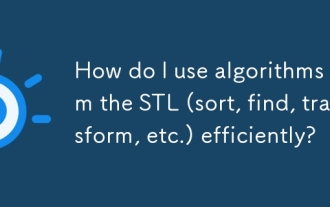
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
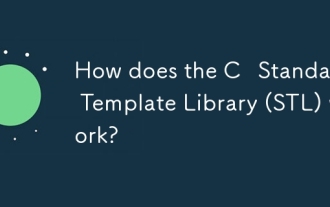
How does the C Standard Template Library (STL) work?
