


How Can I Evaluate Mathematical Expressions Stored as Strings in JavaScript?
Evaluating Mathematical Expressions in JavaScript Strings
Parsing and evaluating mathematical expressions in JavaScript strings poses a challenge. However, there are several libraries and techniques available to tackle this effectively.
One approach involves utilizing the JavaScript Expression Evaluator library. This library enables the evaluation of expressions within strings, as demonstrated in the following example:
Parser.evaluate("2 ^ x", { x: 3 });
Another option is to use the mathjs library, which provides a robust set of mathematical functions. With mathjs, expressions can be evaluated as follows:
math.eval('sin(45 deg) ^ 2');
Other Considerations
Another potential solution proposed in a separate Stack Overflow answer suggests a method for parsing and evaluating expressions manually using regular expressions:
const regex = /([0-9]+)\s*([+\-*\/])\s*([0-9]+)/g; const expression = "1+1"; const result = expression.match(regex).reduce((a, b) => eval(b), 0);
This approach is more intricate but offers greater control over the evaluation process.
Conclusion
By leveraging these libraries or techniques, developers can effectively evaluate mathematical expressions stored as strings, enabling complex calculations and dynamic mathematical operations within JavaScript applications.
The above is the detailed content of How Can I Evaluate Mathematical Expressions Stored as Strings in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




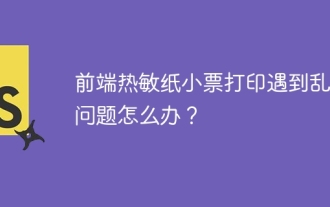
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
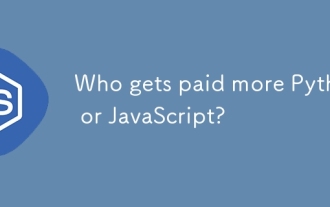
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
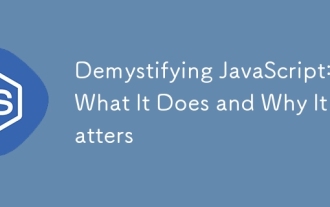
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
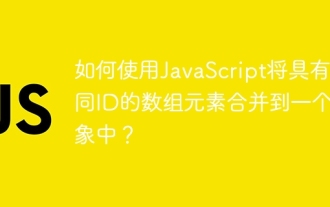
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
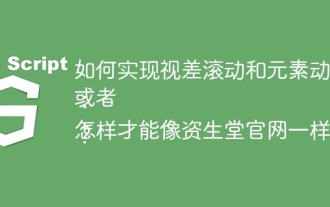
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
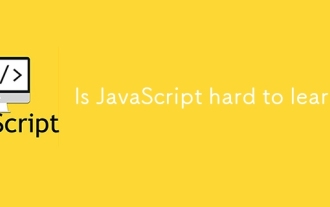
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
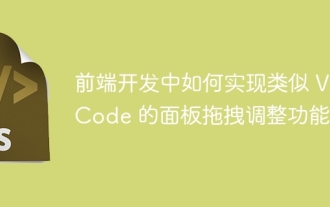
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
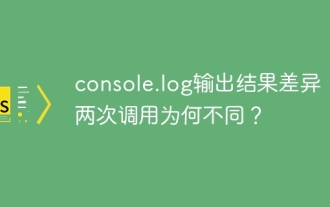
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
