How to Create Idiomatic Iterators in Go?
Creating Idiomatic Iterators in Go
Iterators are essential for iterating over collections in a convenient and expressive manner. While channels may initially seem like a viable option, their push model and memory leakage concerns limit their usefulness.
Idiomatic Approaches
Closures:
Closures offer a straightforward method to implement iterators. By capturing a variable within a function, the function can be used as an iterator, with each call incrementing the variable and returning its value. For instance:
import "fmt" func newEven() func() int { n := 0 return func() int { n += 2 return n } }
Named Types with Methods:
Another approach is to define a named type with a method that iterates over the collection. This method can update its internal state and return the next element in the sequence. For example:
type even int func (e *even) next() int { *e += 2 return int(*e) }
Tradeoffs and Selection:
The choice of approach depends on specific requirements. Channels excel for concurrent scenarios, closures provide flexibility and ease of use, while named types offer clarity and encapsulation.
Chaining Iterators
Functional programming concepts can be leveraged to chain multiple iterators for complex transformations. Functions can be composed into iterators, allowing for operations like mapping, filtering, and folding.
Here's an example of chaining iterators using closures:
import "fmt" type intGen func() int func newEven() intGen { n := 0 return func() int { n += 2 return n } } func mapInt(g intGen, f func(int) int) intGen { return func() int { return f(g()) } } func square(i int) int { return i * i } func main() { gen := mapInt(newEven(), square) fmt.Println(gen()) fmt.Println(gen()) fmt.Println(gen()) }
By utilizing the flexibility and extensibility of Go's function types, iterators can be effortlessly combined to transform and process collections effectively.
The above is the detailed content of How to Create Idiomatic Iterators in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










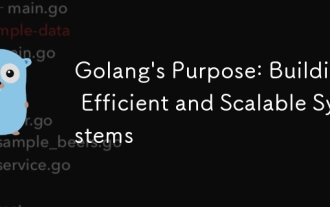
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
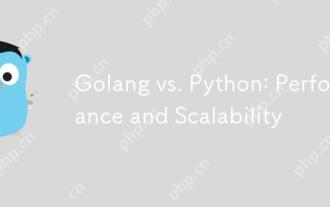
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
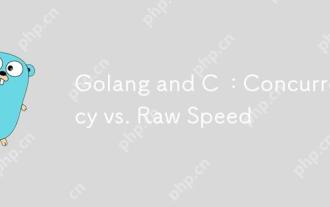
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
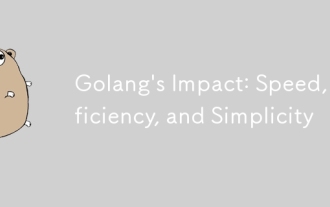
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
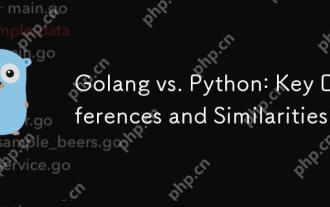
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
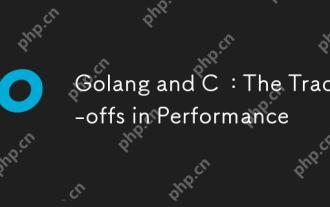
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
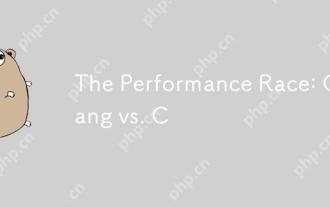
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
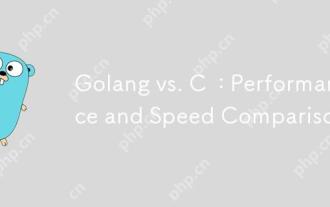
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
