Decorators in TypeScript
Decorators in TypeScript are a powerful feature that allows you to add metadata or modify the behavior of classes, methods, properties or parameters. They are often used in frameworks like Angular to enrich components and services. Whether you're a beginner or an experienced developer, this article guides you step-by-step through creating your own decorators to enhance your TypeScript applications.
Prerequisites
Before you begin, make sure you have the following:
- A basic understanding of TypeScript.
- TypeScript configured with the experimentalDecorators option enabled in your tsconfig.json file:
{ "compilerOptions": { "experimentalDecorators": true } }
What is a decorator?
A decorator is a function applied to a class, method, property or parameter. Preceded by the symbol @, a decorator can modify or enrich the element to which it is attached. Its main uses include:
- Add metadata: Useful for providing additional information about a class or property.
- Modify behavior: allows you to dynamically change the operation of a method or class.
- Injecting dependencies: practical in modular architectures.
Creating a Component Decorator
Step 1: Define the Decorator
We will create a decorator that adds a componentName property to a class.
function Component(name: string) { return function (constructor: Function) { constructor.prototype.componentName = name; }; }
Explanation:
This decorator receives a name string and adds it as a property on the class prototype. All instances of this class will have access to this property.
Step 2: Apply Decorator
Let's apply the decorator to a class.
@Component('MonComposant') class MonComposant { constructor() { console.log(`Le nom du composant est : ${this.componentName}`); } }
Step 3: Test the Decorator
Let's create an instance of the class to check how it works.
const composant = new MonComposant(); // Affiche : Le nom du composant est : MonComposant
Creating an Input Decorator
Step 1: Define the Input Decorator
This decorator monitors and logs changes to a property.
function Input() { return function (target: any, propertyKey: string) { let value: any; const getter = () => { return value; }; const setter = (newValue: any) => { console.log(`La valeur de ${propertyKey} a été mise à jour : ${newValue}`); value = newValue; }; Object.defineProperty(target, propertyKey, { get: getter, set: setter, enumerable: true, configurable: true, }); }; }
Explanation:
The decorator uses Object.defineProperty to intercept changes to the property. This allows you to add custom logic, such as change logging.
Step 2: Apply the Input Decorator
Let's apply it to a property.
{ "compilerOptions": { "experimentalDecorators": true } }
Step 3: Test the Input Decorator
Change the property to observe the effect.
function Component(name: string) { return function (constructor: Function) { constructor.prototype.componentName = name; }; }
Conclusion
TypeScript decorators provide an elegant and powerful way to add functionality and metadata to your classes and properties. By following this article, you have learned to:
- Create a component decorator to enrich a class.
- Implement an input decorator to monitor property changes.
These simple examples show how decorators can improve the readability and maintainability of your code. Explore more of the official TypeScript documentation to discover even more advanced applications, like using reflected metadata with Reflect.metadata.
The above is the detailed content of Decorators in TypeScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










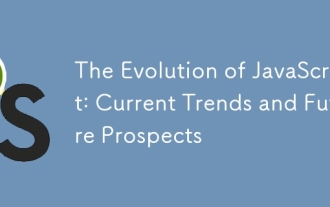
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
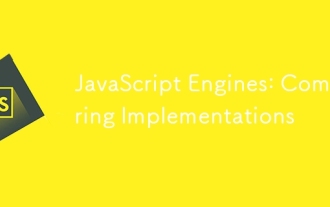
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
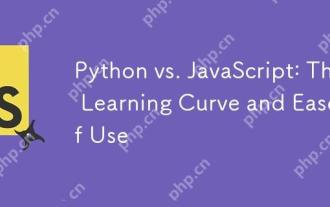
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
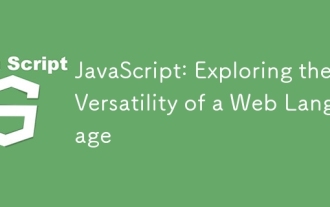
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
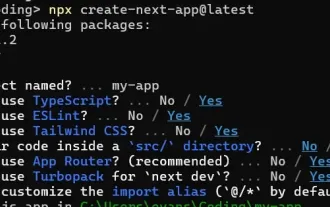
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
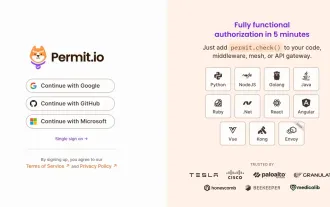
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
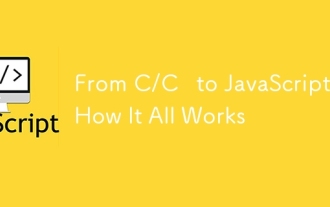
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
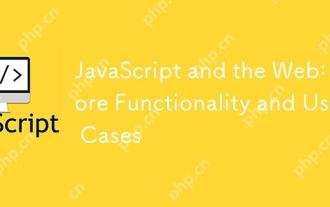
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
