


How Can I Read a Text File into a Single String Variable Without Newlines?
Reading a Text File into a String Variable Without Newlines
When working with text files, you may encounter the need to read the contents into a string variable while excluding the newline characters. This is particularly useful when you want to store the entire file's content as a single string.
Reading the File Using 'with' and 'replace()'
To accomplish this, one approach is to use a 'with' statement to open the file and read its contents into a string. Once you have the contents as a string, you can use the 'replace()' method to remove all occurrences of the newline character ('n'). Here's an example:
with open('data.txt', 'r') as file: data = file.read().replace('\n', '')
In this example, the 'with' statement ensures that the file is closed properly after use. The 'file.read()' method reads the entire contents of the file into a string. Finally, the 'replace()' method replaces every newline character with an empty string, effectively removing them.
Alternative Method with 'rstrip()'
If you know for certain that the file contains only one line of text, you can simplify the process by using the 'rstrip()' method. This method removes any trailing whitespace characters, including newlines, from the string.
with open('data.txt', 'r') as file: data = file.read().rstrip()
By using these techniques, you can effectively read a text file into a string variable and strip all unwanted newline characters, allowing you to work with the contents as a single-line string.
The above is the detailed content of How Can I Read a Text File into a Single String Variable Without Newlines?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


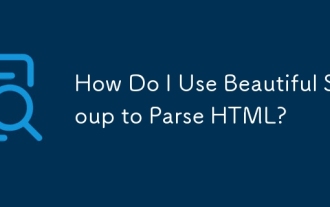
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
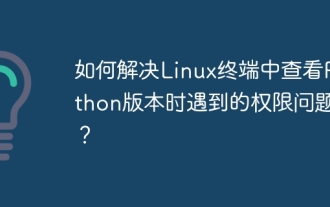
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
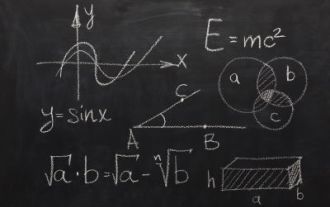
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
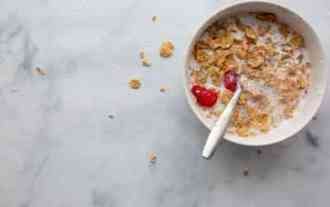
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
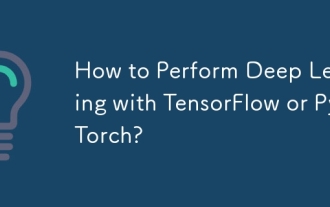
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap

This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
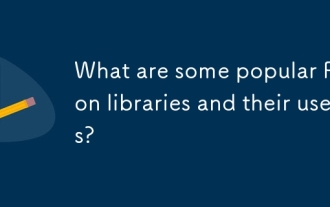
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
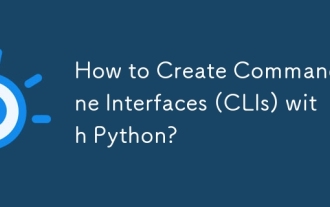
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
