How Can I Serve Static Files Embedded in a Go Binary?
Serving Static Files from Binary in Go: A Custom FileSystem
When serving static files in Go, the FileServer handler simplifies the process. However, for cases where only a few static files need to be deployed, an alternative approach can eliminate the need for managing those files externally.
InMemoryFS Implementation
The FileServer requires a FileSystem object, leading to the question of how to bake static files into a binary. An implementation of InMemoryFS can serve files from memory, eliminating the need to interact with the file system directly.
package main import ( "io" "net/http" "time" ) type InMemoryFS map[string]io.ReadCloser // Implements FileSystem interface func (fs InMemoryFS) Open(name string) (http.File, error) { f, ok := fs[name] if !ok { return nil, os.ErrNotExist } return &InMemoryFile{ ReadCloser: f, }, nil } type InMemoryFile struct { io.ReadCloser } // Implements http.File interface func (f *InMemoryFile) Close() error { return nil } func (f *InMemoryFile) Stat() (os.FileInfo, error) { fi, err := f.ReadCloser.Stat() if err != nil { return nil, err } return &InMemoryFileInfo{ name: f.ReadCloser.(os.FileInfo).Name(), size: fi.Size(), modTime: fi.ModTime(), }, nil } type InMemoryFileInfo struct { name string size int64 modTime time.Time } // Implements os.FileInfo func (s *InMemoryFileInfo) Name() string { return s.name } func (s *InMemoryFileInfo) Size() int64 { return s.size } func (s *InMemoryFileInfo) Mode() os.FileMode { return 0644 } func (s *InMemoryFileInfo) ModTime() time.Time { return s.modTime } func (s *InMemoryFileInfo) IsDir() bool { return false } func (s *InMemoryFileInfo) Sys() interface{} { return nil }
Example Usage
The InMemoryFS implementation can be utilized with the FileServer as follows:
func main() { FS := make(InMemoryFS) // Load static files into memory FS["foo.html"] = os.Open("foo.html") FS["bar.css"] = os.Open("bar.css") http.Handle("/", http.FileServer(FS)) http.ListenAndServe(":8080", nil) }
Alternative Considerations
Instead of creating a custom FileSystem, it might be simpler to rewrite the serving part to handle a small number of static files directly, avoiding the need to emulate a complete file system. Ultimately, the best approach depends on the specific requirements of the project.
The above is the detailed content of How Can I Serve Static Files Embedded in a Go Binary?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










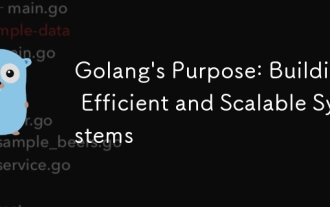
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
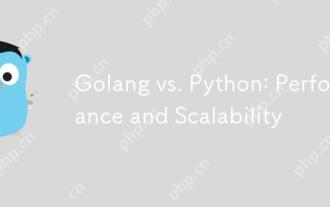
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
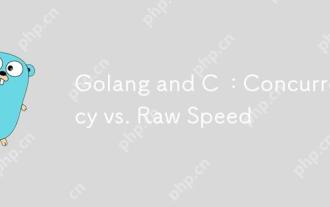
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
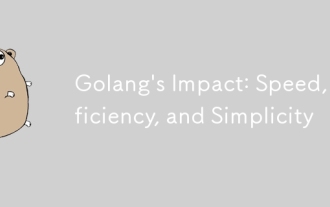
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
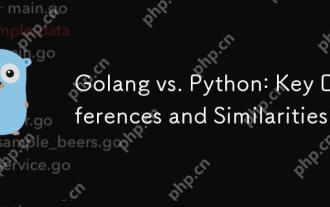
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
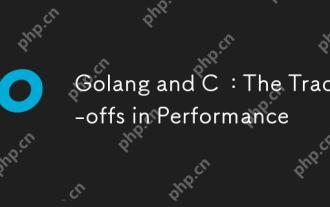
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
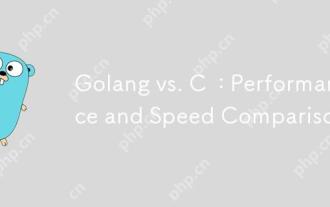
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
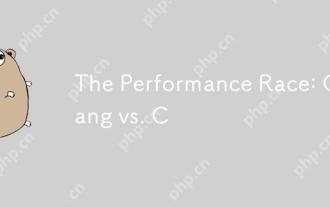
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
