


How Can I Serialize a Lambda Expression in Java Without Creating a Dummy Interface?
Dec 07, 2024 am 09:13 AMSerializing a Lambda: A Solution Without Dummy Interfaces
In Java programming, it's not uncommon to encounter scenarios where you need to serialize lambda expressions. However, attempts to directly serialize a lambda often result in a NotSerializableException.
Consider the following example:
public static void main(String[] args) throws Exception { File file = Files.createTempFile("lambda", "ser").toFile(); try (ObjectOutput oo = new ObjectOutputStream(new FileOutputStream(file))) { Runnable r = () -> System.out.println("Can I be serialized?"); oo.writeObject(r); } try (ObjectInput oi = new ObjectInputStream(new FileInputStream(file))) { Runnable r = (Runnable) oi.readObject(); r.run(); } }
In this case, serializing the lambda throws a NotSerializableException.
To overcome this challenge, Java 8 introduces a clever solution: casting an object to an intersection of types. By adding multiple bounds to the lambda's type, you can elegantly make it serializable:
Runnable r = (Runnable & Serializable)() -> System.out.println("Serializable!");
This technique allows the lambda to automatically inherit the Serializable interface, enabling it to be serialized without the need for a "dummy" SerializableRunnable interface.
The above is the detailed content of How Can I Serialize a Lambda Expression in Java Without Creating a Dummy Interface?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
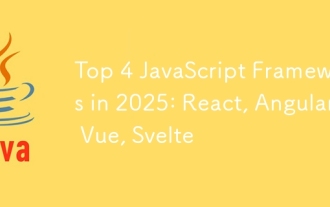
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
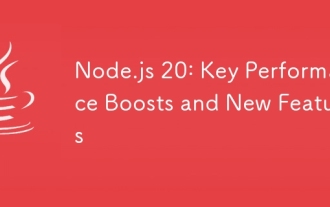
Node.js 20: Key Performance Boosts and New Features
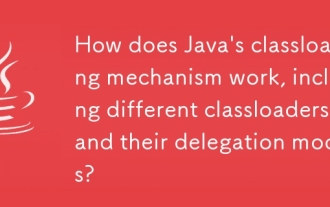
How does Java's classloading mechanism work, including different classloaders and their delegation models?
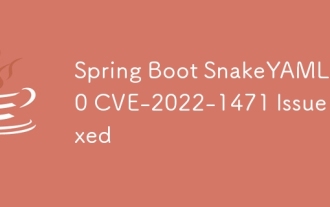
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
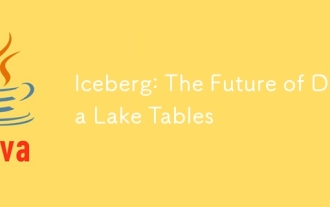
Iceberg: The Future of Data Lake Tables
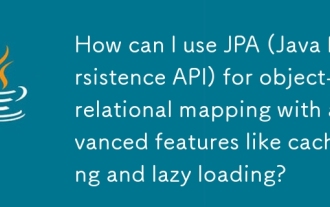
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
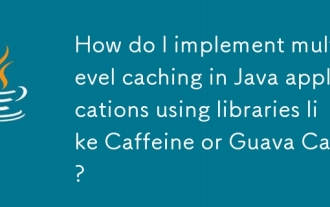
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
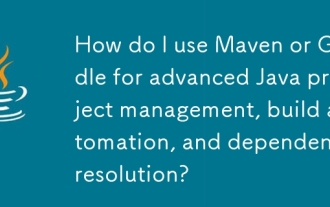
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
