


How to Implement a Loading Screen During Route Transitions in Angular?
Displaying a Loading Screen During Route Transitions in Angular 2
Angular Router offers Navigation Events that enable you to monitor changes and modify the UI accordingly. These events include NavigationStart, NavigationEnd, NavigationCancel, and NavigationError.
Using Navigation Events
To display a loading screen during route changes, you can subscribe to these Navigation Events within your root component (typically app.component.ts) and toggle the loading UI based on the specific event triggered.
First, import the necessary event related classes from the @angular/router and define a boolean flag, loading, within your root component.
import { Router, Event as RouterEvent, NavigationStart, NavigationEnd, NavigationCancel, NavigationError } from '@angular/router' @Component({}) export class AppComponent { loading = true; // Initializing to true to show loading spinner on first load constructor(private router: Router) { this.router.events.subscribe((e : RouterEvent) => { this.navigationInterceptor(e); }) } }
Within the navigationInterceptor method, you can listen for the NavigationStart, NavigationEnd, NavigationCancel, and NavigationError events and toggle the loading flag based on each event.
// Shows and hides the loading spinner during RouterEvent changes navigationInterceptor(event: RouterEvent): void { if (event instanceof NavigationStart) { this.loading = true; } if (event instanceof NavigationEnd) { this.loading = false; } if (event instanceof NavigationCancel) { this.loading = false; } if (event instanceof NavigationError) { this.loading = false; } }
Within your root template, you can then use conditional rendering to display the loading overlay based on the loading flag's state.
<div>
By following this approach, you can effectively display a loading screen during route transitions in your Angular 2 application.
Performance Optimization
If performance is a concern, you can leverage NgZone and Renderer to enhance the loading spinner's performance, as shown in the updated code snippet below:
private _navigationInterceptor(event: RouterEvent): void { if (event instanceof NavigationStart) { this.ngZone.runOutsideAngular(() => { this.renderer.setElementStyle( this.spinnerElement.nativeElement, 'opacity', '1' ) }) } if (event instanceof NavigationEnd) { this._hideSpinner() } // Set loading state to false in both of the below events to // hide the spinner in case a request fails if (event instanceof NavigationCancel) { this._hideSpinner() } if (event instanceof NavigationError) { this._hideSpinner() } } private _hideSpinner(): void { this.ngZone.runOutsideAngular(() => { this.renderer.setElementStyle( this.spinnerElement.nativeElement, 'opacity', '0' ) }) }
This technique bypasses Angular's change detection mechanism when updating the spinner's opacity, resulting in smoother transitions.
The above is the detailed content of How to Implement a Loading Screen During Route Transitions in Angular?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










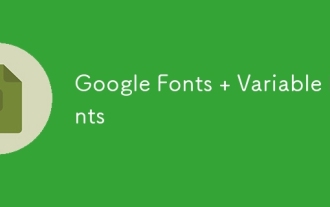
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
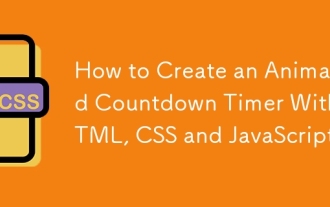
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
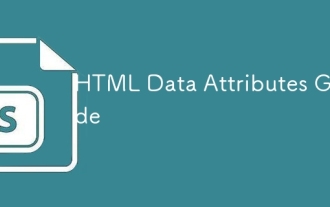
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
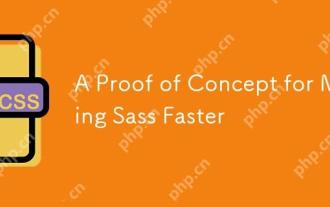
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
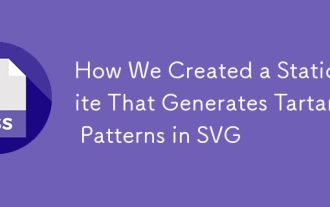
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
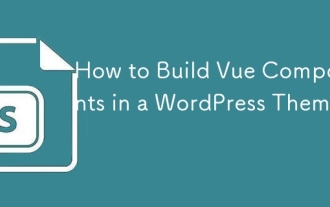
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
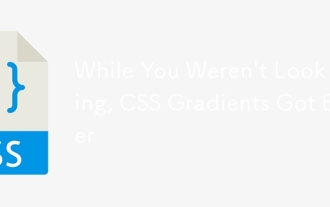
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
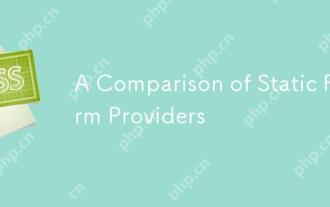
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
