How to Smoothly Rotate an Image 90 Degrees in Java Swing Using a Timer?
How to rotate an image gradually in Swing?
This question concerns an issue with rotating an image when a button is clicked in Java Swing. The goal is to have the image rotate smoothly to 90 degrees.
Question Details:
The provided code contains several classes: RotateButtonSSCE, CrossingPanelSSCE, VisualizationPanelSSCE, and a main method in TestGUISSCE. The RotateButtonSSCE class creates a button that triggers rotation, the CrossingPanelSSCE class includes the image that needs to be rotated, and the VisualizationPanelSSCE class provides a surface for drawing the image.
The issue arises when trying to rotate the image gradually. The code attempts to achieve this using a timer that calls a method to rotate the image incrementally, but it doesn't seem to work as intended.
Answer:
In addition to the corrections suggested by @tulskiy, it's important to note that the given code has some structural issues that may contribute to the problem. Here are key observations and recommendations:
- Use top-level or nested classes: An SSCCE (Short, Self Contained, Correct (Compilable), Example) should be easy to understand without requiring the creation of multiple public classes. Consider using top-level (package-private) or nested classes for convenience.
- Construct GUI on the event dispatch thread: Always create your GUI on the event dispatch thread to avoid threading issues.
- Utilize the paintComponent method: In this example, the paintComponent() method in CrossingPanelSSCE is responsible for displaying the image. To rotate the image gradually, you can use the AffineTransform class to alter the graphics context's transform. By applying appropriate rotations and translations, you can achieve a smooth rotation effect.
Revised Code Excerpt:
Here's a revised portion of the CrossingPanelSSCE class that rotates the image using AffineTransform:
import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.geom.AffineTransform; import java.awt.image.BufferedImage; import javax.swing.*; public class CrossingPanelSSCE extends JPanel { // ... other code private BufferedImage image; private double rotationAngle = 0; private AffineTransform transform = new AffineTransform(); // ... other methods @Override public void paintComponent(Graphics g) { super.paintComponent(g); Graphics2D g2d = (Graphics2D) g; // Apply the rotation transform to the graphics context g2d.setTransform(transform); // Draw the image at its center g2d.drawImage(image, -image.getWidth() / 2, -image.getHeight() / 2, null); } public void rotateImage(double angle) { rotationAngle += angle; // Update the AffineTransform with the new rotation angle transform.rotate(Math.toRadians(angle), image.getWidth() / 2, image.getHeight() / 2); // Repaint the panel to display the updated image repaint(); } }
Usage in RotateButtonSSCE (Button ActionListener):
public void actionPerformed(ActionEvent ev) { vis.rotateImage(ROTATE_ANGLE_OFFSET); }
This code increments the rotation angle by a small value (ROTATE_ANGLE_OFFSET) on each button click and updates the image accordingly. You can adjust the ROTATE_ANGLE_OFFSET to control the speed of the rotation animation.
The above is the detailed content of How to Smoothly Rotate an Image 90 Degrees in Java Swing Using a Timer?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
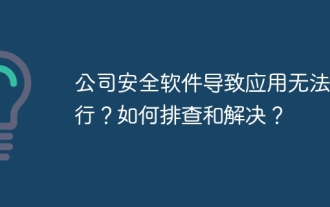
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
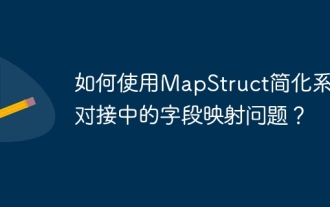
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
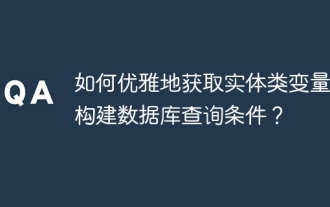
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
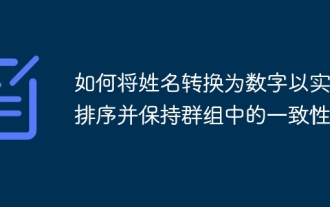
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
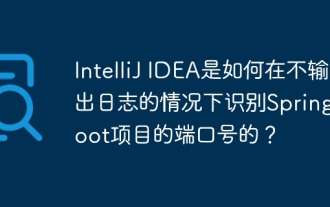
Start Spring using IntelliJIDEAUltimate version...
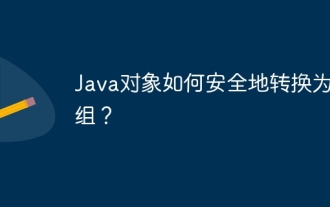
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
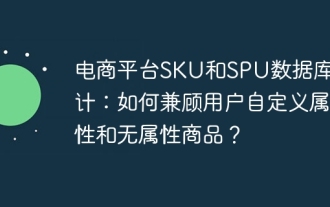
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
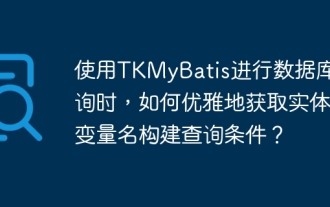
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
