


Why Does My Go Code Print 'Three' Three Times Instead of 'One,' 'Two,' and 'Three' in a Goroutine Data Race?
Understanding the Curious Behavior of Goroutines in a Data Race Scenario
In the provided Go code, we create a slice of struct fields called data containing names "one," "two," and "three." The code iterates through the slice, creating goroutines to print each field's name using the print method. However, contrary to expectations, the code repeatedly prints "three" three times, rather than the intended sequence of "one," "two," and "three."
Unveiling the Data Race
This curious behavior stems from a data race, which occurs when multiple goroutines access and potentially modify the same shared data concurrently. In this case, the issue arises from the implicit use of the address of the range variable v when creating the goroutines. When the loop variable v is changed in each iteration, the goroutines end up using its final value, resulting in the constant printing of "three."
Addressing the Data Race
To resolve this issue, we can adopt several approaches:
-
Creating a New Variable in Each Loop Iteration: Within the loop, we can declare a new variable with the same name as the range variable, effectively creating a new scope for the variable.
for _, v := range data { v := v // Declare a new variable `v` within the loop scope. go v.print() }
Copy after login -
Using a Slice of Pointers: Instead of using a slice of struct fields, we can use a slice of pointers to the fields. This ensures that the goroutines receive pointers to individual field elements, preventing data race issues.
data := []*field{ {"one"},{"two"},{"three"} } for _, v := range data { go v.print() }
Copy after login -
Passing the Address of the Slice Element: Another alternative is to pass the address of each element in the slice to the goroutine.
for i := range data { v := &data[i] // Take the address of the slice element. go v.print() }
Copy after login -
Using Anonymous Functions and Passing Range Variables as Arguments: If the goroutine function is within an anonymous function, we can avoid the issue by passing the range variables as arguments to the function.
for _, v := range data { go func(v field) { // Pass the range variable `v` as an argument. v.print() }(v) }
Copy after login
These approaches ensure that the goroutines have their own copies of the data they need, eliminating the data race and producing the correct output of "one," "two," and "three" in any order.
The above is the detailed content of Why Does My Go Code Print 'Three' Three Times Instead of 'One,' 'Two,' and 'Three' in a Goroutine Data Race?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










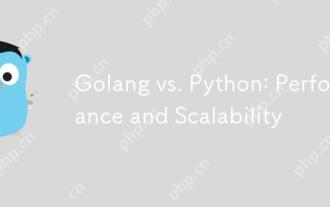
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
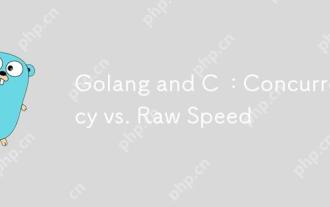
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
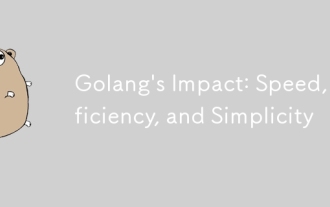
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
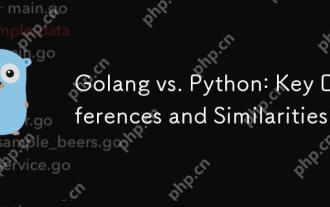
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
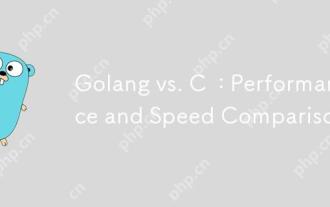
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
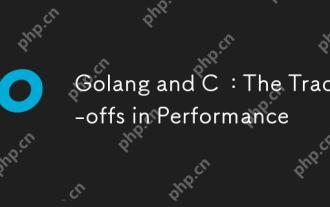
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
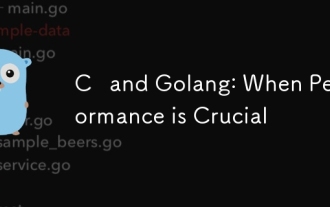
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
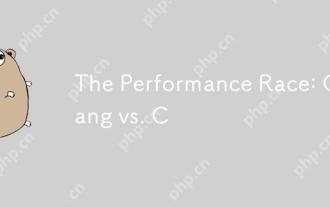
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
