How to Mock Void Methods in Mockito?
Mocking Void Methods with Mockito
How can one mock methods with a void return type using the Mockito framework? This particular question arises frequently in scenarios where an object method does not return a value but instead performs an action.
Overcoming the Challenge
The Mockito API offers a range of options to handle this situation, including methods like doThrow(), doAnswer(), doNothing(), and doReturn().
- doThrow(Exception): Throws a predefined Exception when the mock method is invoked.
- doAnswer(Answer): Allows for defining custom behavior when the mock method is called. The Answer interface accepts an InvocationOnMock object and returns an appropriate value (Void in this case).
Example Implementation
Consider the following example scenario:
public class World { private String state; // Setter for state public void setState(String s) { this.state = s; } } public class WorldTest { @Test public void testingWorld() { World mockWorld = mock(World.class); // Mock the setState method using doAnswer doAnswer(new Answer<Void>() { @Override public Void answer(InvocationOnMock invocation) { Object[] args = invocation.getArguments(); System.out.println("Called with arguments: " + Arrays.toString(args)); return null; } }).when(mockWorld).setState(anyString()); // Call the mock method and observe the output mockWorld.setState("Mocked"); } }
By employing the doAnswer method, we can specify custom behavior for the mock setState method. In the example above, we print the arguments passed to the method, providing visibility into its invocation.
The above is the detailed content of How to Mock Void Methods in Mockito?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










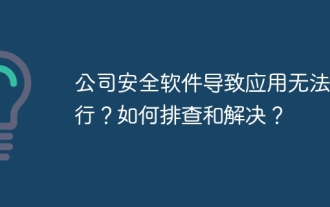
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
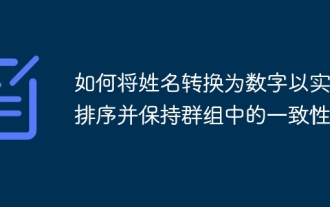
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
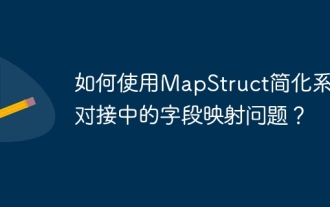
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
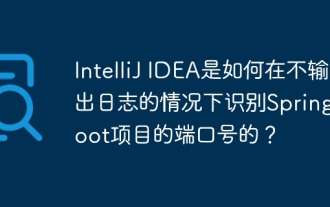
Start Spring using IntelliJIDEAUltimate version...
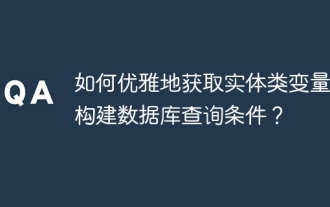
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
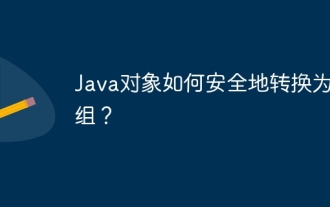
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
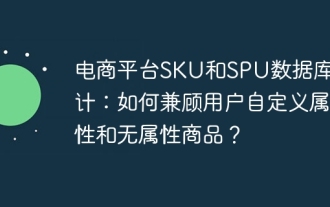
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
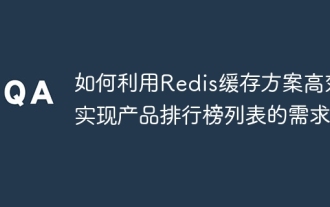
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
