


How Can I Detect Clicks Inside Cross-Domain Iframes Using JavaScript?
Detecting Clicks Within iframes Using JavaScript
If you're looking for a way to determine whether a user has clicked within an iframe, you might have stumbled upon the limitation that cross-domain iframes cannot be monitored directly in JavaScript. Nonetheless, there's a clever technique that can help you achieve your goal.
The solution lies in overlaying an invisible div over the iframe. When a click occurs within the iframe, it will also trigger the click event for the overlaying div. By listening for this event, you can indirectly detect a click within the iframe.
Here's a code example to illustrate this approach:
const message = document.getElementById("message"); // Ensure the main document has focus to register the blur event. window.focus(); window.addEventListener("blur", () => { setTimeout(() => { if (document.activeElement.tagName === "IFRAME") { message.textContent = "clicked " + Date.now(); console.log("clicked"); } }); }, { once: true });
<div>
In this example, the message div is used to display "clicked" along with the timestamp when a click occurs within the iframe. Note that this approach works effectively in Chrome, Firefox, and IE 11 (and likely other browsers as well). It provides a straightforward solution to monitor user interaction within cross-domain iframes, especially when you have no control over the iframe tags used.
The above is the detailed content of How Can I Detect Clicks Inside Cross-Domain Iframes Using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


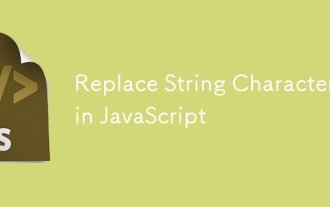
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
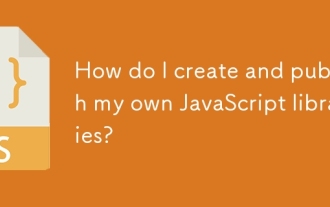
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
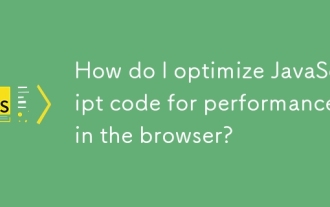
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
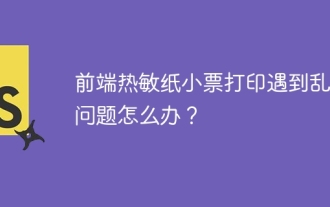
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
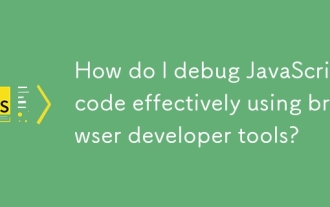
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
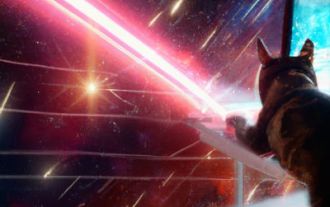
This article outlines ten simple steps to significantly boost your script's performance. These techniques are straightforward and applicable to all skill levels. Stay Updated: Utilize a package manager like NPM with a bundler such as Vite to ensure
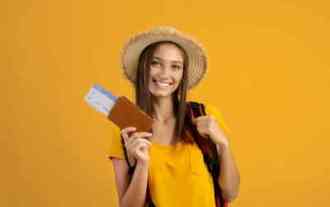
Sequelize is a promise-based Node.js ORM. It can be used with PostgreSQL, MySQL, MariaDB, SQLite, and MSSQL. In this tutorial, we will be implementing authentication for users of a web app. And we will use Passport, the popular authentication middlew
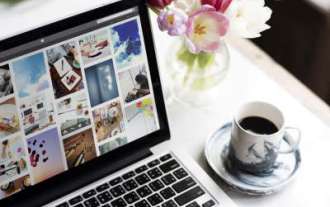
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
