How Can I Dynamically Assign JavaScript Variables Using Strings?
Converting Strings to Variable Names in JavaScript
When passing strings into a function, developers often encounter the challenge of setting the corresponding variable within the function. This article presents a solution using the window object to dynamically access global variables by their string names.
Specifically, to set the variable onlyVideo within the function, the following code can be used:
window["onlyVideo"] = something;
This approach allows the dynamic assignment of variables based on strings, eliminating the need for hard-coded if statements.
As an alternative, JavaScript objects provide a more robust mechanism for managing and accessing properties by their string names. A simple example demonstrates how to create and manipulate JavaScript objects:
// create JavaScript object var obj = { "key1": 1 }; // assign - set "key2" to 2 obj.key2 = 2; // read values console.log(obj.key1 === 1); console.log(obj.key2 === 2); // read values with a string, same result as above // but works with special characters and spaces // and of course variables console.log(obj["key1"] === 1); console.log(obj["key2"] === 2); // read with a variable var key1Str = "key1"; console.log(obj[key1Str] === 1);
The above is the detailed content of How Can I Dynamically Assign JavaScript Variables Using Strings?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


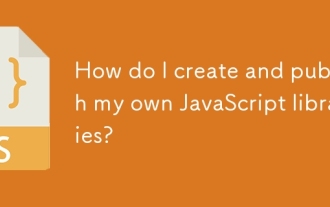
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
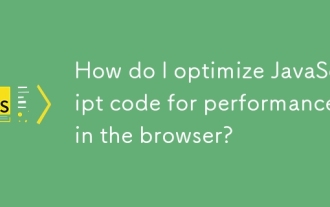
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
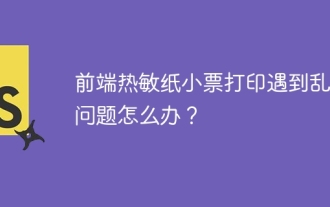
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
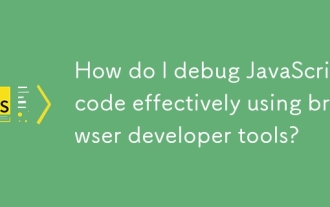
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
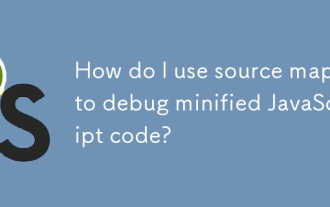
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
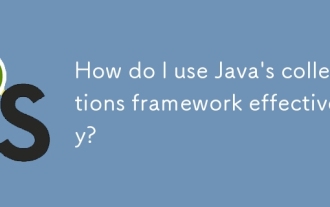
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
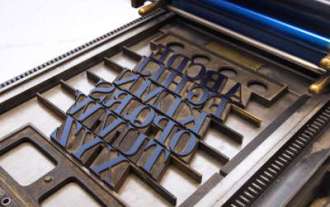
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
