How to Effectively Pass Arguments to Gin Router Handlers?
How to Pass Arguments to Router Handlers in Gin Web Framework?
When building a RESTful API with Gin, passing arguments to router handlers becomes essential, especially for sharing common resources like database connections. While storing arguments in global variables may seem straightforward, there are better options available.
Closures for Explicit Passing
A preferred method is using closures to pass dependencies explicitly. By encapsulating handler code in a closure, you can return a function that satisfies Gin's HandlerFunc interface.
// SomeHandler returns a gin.HandlerFunc to satisfy Gin's router methods. func SomeHandler(db *sql.DB) gin.HandlerFunc { fn := func(c *gin.Context) { // Handler code goes here. For example: rows, err := db.Query(...) c.String(200, results) } return gin.HandlerFunc(fn) } func main() { db, err := sql.Open(...) // Handle error router := gin.Default() router.GET("/test", SomeHandler(db)) router.Run(":8080") }
This approach allows you to explicitly pass the database connection to the handler function while maintaining separation of concerns.
Global Variables
While global variables may seem tempting for small projects, it's generally discouraged. It can lead to tightly coupled code and make it harder to maintain and test your application. If you do choose to use global variables, ensure they encapsulate dependencies in a clear and well-structured manner.
Optional Function Arguments
Go does not support optional function arguments natively. However, you can use functional programming techniques, such as returning a function from a function, to achieve similar functionality. This approach can provide flexibility and code reuse.
The above is the detailed content of How to Effectively Pass Arguments to Gin Router Handlers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










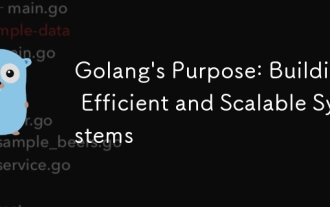
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
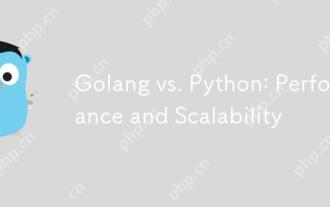
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
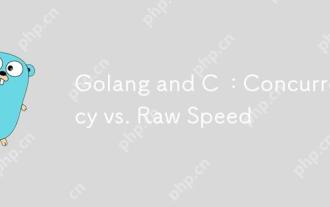
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
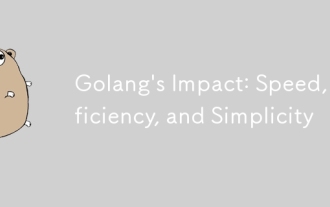
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
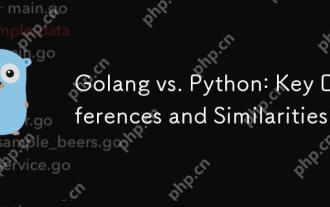
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
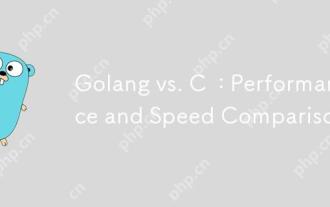
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
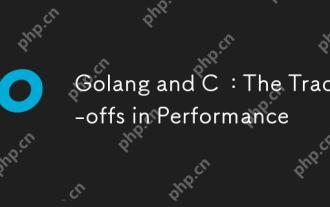
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
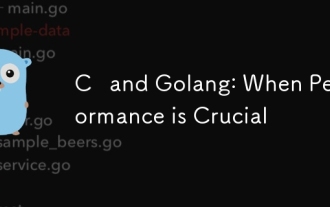
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
