


How to Extract Multiple Text Snippets Enclosed in Square Brackets Using PHP?
Capturing Multiple Text Snippets within Square Brackets in PHP
To extract multiple text segments enclosed within square brackets, a regular expression can be utilized. Here's how to achieve this in PHP:
Firstly, the regular expression /[.*?]/ is not suitable for capturing multiple instances. Instead, to match all strings with brackets, you can use the regex:
$text = '[This] is a [test] string, [eat] my [shorts].'; preg_match_all("/\[[^\]]*\]/", $text, $matches); var_dump($matches[0]);
If you wish to extract the text within the brackets without the brackets themselves, use the regex:
$text = '[This] is a [test] string, [eat] my [shorts].'; preg_match_all("/\[([^\]]*)\]/", $text, $matches); var_dump($matches[1]);
Alternatively, a slightly slower but equivalent option is to use the regex:
$text = '[This] is a [test] string, [eat] my [shorts].'; preg_match_all("/\[(.*?)\]/", $text, $matches); var_dump($matches[1]);
By employing these variations, you can capture multiple text segments within square brackets based on your specific requirements and string content.
The above is the detailed content of How to Extract Multiple Text Snippets Enclosed in Square Brackets Using PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


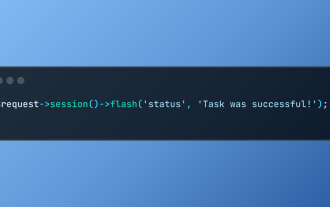
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
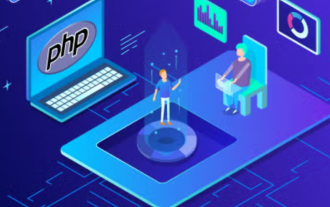
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
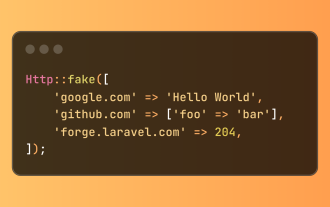
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
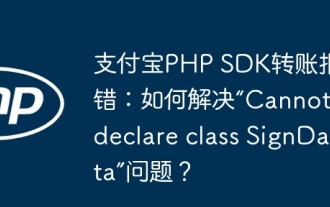
Alipay PHP...
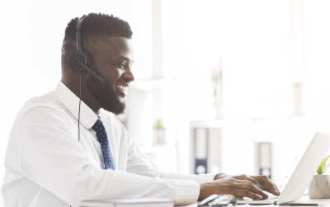
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
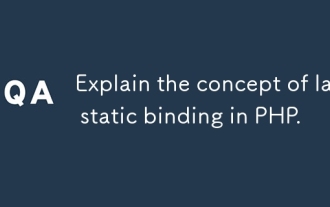
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
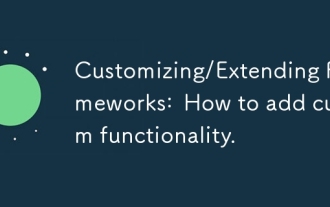
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
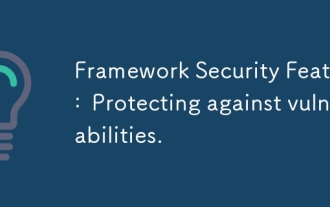
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
