


How Can I Efficiently Convert a Python List of Alternating Keys and Values into a Dictionary?
Converting [key1,val1,key2,val2] Lists to Dictionaries in Python
In Python, it is common to encounter lists where each pair of elements maps to a key-value pair in a dictionary. To convert such lists to dictionaries, the most syntactically elegant approach is to utilize the built-in zip() function in conjunction with dictionary construction.
For instance, given a list a with alternating keys and values:
a = ['hello','world','1','2']
We can efficiently create a dictionary b where each key from a maps to its corresponding value as follows:
b = dict(zip(a[::2], a[1::2]))
Here, a[::2] extracts the even elements, representing keys, and a[1::2] extracts the odd elements, representing values. The dict() constructor then combines these lists into a dictionary.
For larger lists, a memory-efficient alternative is to leverage the itertools.izip() function, which iteratively consumes the elements from a in pairs:
from itertools import izip i = iter(a) b = dict(izip(i, i))
In Python 3, a similar result can be achieved using a dict comprehension along with range():
b = {a[i]: a[i+1] for i in range(0, len(a), 2)}
Alternatively, the zip() function can be used directly, as it has been lazy in Python 3:
i = iter(a) b = dict(zip(i, i))
For brevity, the "walrus" operator (:=) can be employed in Python 3.8 and later to perform assignment in a single line:
b = dict(zip(i := iter(a), i))
The above is the detailed content of How Can I Efficiently Convert a Python List of Alternating Keys and Values into a Dictionary?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


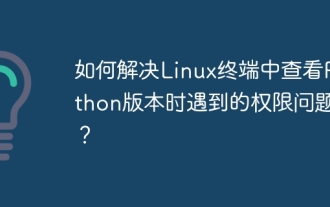
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
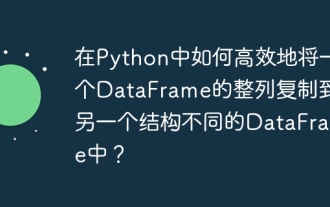
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
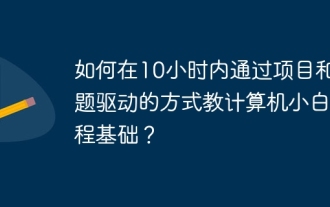
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
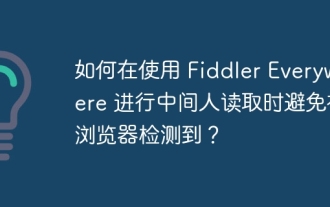
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
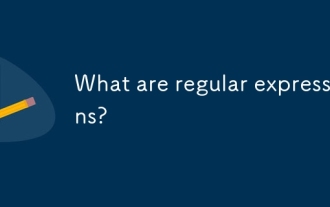
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
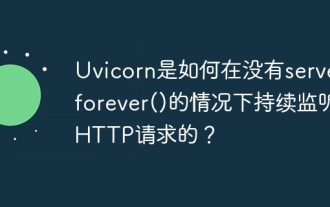
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
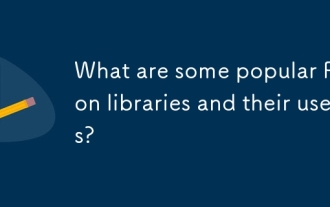
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
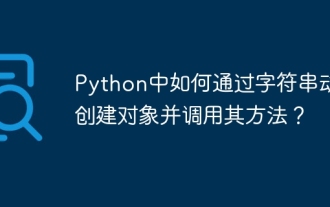
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
