Securing Your Node.js Application: A Comprehensive Guide
In today's digital landscape, securing your Node.js application is paramount. From global leaders like Netflix and Uber, to startups building the next big thing, Node.js powers some of the most demanding and high-performance applications. However, vulnerabilities in your application can lead to unauthorized access, data breaches, and a loss of user trust.
This guide combines practical security practices with key concepts from the OWASP Web Security Testing Guide (WSTG) to help you fortify your Node.js application. Whether you're managing real-time operations or scaling to millions of users, this comprehensive resource will ensure your application remains secure, reliable, and resilient.
Information Gathering (WSTG-INFO)
Information Gathering is often the first step an attacker takes to learn more about your application. The more information they can collect, the easier it becomes for them to identify and exploit vulnerabilities.
Typical Express.js Server Configuration and Fingerprinting
By default, Express.js includes settings that can inadvertently reveal information about your server. A common example is the X-Powered-By HTTP header, which indicates that your application is using Express.
Example Vulnerable Code:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this setup, every HTTP response includes the X-Powered-By: Express header.
Issue:
- Fingerprinting: Attackers can use this header to determine the technologies you're using. Knowing you're running Express allows them to tailor attacks to known vulnerabilities in specific versions of Express or Node.js.
Mitigation:
Disable this header to make it harder for attackers to fingerprint your server.
Improved Code:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Enhanced Mitigation with Helmet:
A better approach is to use the helmet middleware, which sets various HTTP headers to improve your app's security.
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Why Use Helmet?
- Comprehensive Security Headers: Helmet sets multiple HTTP headers that help protect your app from well-known web vulnerabilities.
- Ease of Use: With just one line, you enhance your application's security posture significantly.
Configuration and Deployment Management Testing (WSTG-CONF)
Configuration and deployment management are critical aspects of application security. Misconfigurations can serve as open doors for attackers.
Running in Development Mode in Production
Running your application in development mode on a production server can expose detailed error messages and stack traces.
Example Vulnerable Code:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this setup, detailed error messages are sent to the client.
Issue:
- Information Leakage: Detailed error messages and stack traces can reveal sensitive information about your application's structure, dependencies, and file paths.
- Facilitates Exploitation: Attackers can use this information to identify potential vulnerabilities and craft targeted attacks.
Mitigation:
Set NODE_ENV to 'production' and use generic error messages in production.
Improved Code:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Best Practices:
- Set Environment Variables Correctly: Ensure that NODE_ENV is set to 'production' in your production environment.
- Internal Logging: Log errors internally for debugging purposes without exposing details to the end-user.
Using Default or Weak Credentials
Using default or weak credentials, such as a simple secret key for signing JSON Web Tokens (JWTs), is a common security mistake.
Example Vulnerable Code:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Issue:
- Weak Secret Key: Using a simple or common string like 'secret' makes it easy for attackers to guess or brute-force the key.
- Hard-Coded Secrets: Storing secrets directly in your code increases the risk of exposure if your codebase is compromised.
- Token Forgery: Attackers who know your secret key can forge valid JWTs, gaining unauthorized access.
Mitigation:
Use a strong, secure secret key and store it securely.
Improved Code:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
Best Practices:
- Environment Variables: Do not commit secrets to version control. Use environment variables or configuration files that are not checked into source control.
- Rotate Secrets: Implement a process to rotate secrets periodically.
- Validate Configuration: Ensure that all required environment variables are set during application startup.
Identity Management Testing (WSTG-IDNT)
Identity management is crucial for protecting user accounts and preventing unauthorized access.
Weak Username Policies and Account Enumeration
Allowing weak usernames and providing specific error messages can lead to account enumeration attacks.
Example Vulnerable Code:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Issue:
- Weak Usernames: Allowing short or simple usernames increases the risk of account compromise.
- Account Enumeration: Specific error messages can help attackers determine valid usernames.
Mitigation:
Implement username validation and use generic error messages.
Improved Code:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Username Validation: Ensures usernames meet specific criteria, reducing weak entries.
- Generic Error Messages: Prevent attackers from identifying valid usernames through error responses.
Authentication Testing (WSTG-ATHN)
Authentication mechanisms are vital for verifying user identities and preventing unauthorized access.
Brute-Force Attacks on Passwords and 2FA
Lack of protections allows attackers to guess passwords or 2FA codes through repeated attempts.
Example Vulnerable Code:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Issue:
- Unlimited Login Attempts: Attackers can repeatedly try different passwords or 2FA codes.
- Weak 2FA Implementation: Static or predictable 2FA codes are vulnerable.
Mitigation:
Implement rate limiting and enhance 2FA security.
Improved Code:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
Additional Measures:
- Use CAPTCHA After Failed Attempts: Introduce CAPTCHA after several failed login attempts to verify human users.
- Employ TOTP for 2FA: Use time-based one-time passwords for dynamic and secure 2FA codes.
Explanation:
- Rate Limiting: Reduces automated attack risks by limiting login attempts.
- Enhanced 2FA: Time-based codes improve security over static codes.
Authorization Testing (WSTG-ATHZ)
Authorization ensures users access only the resources they are permitted to use, preventing unauthorized actions.
Insecure Direct Object References (IDOR)
Users can access unauthorized resources by manipulating identifiers in requests.
Example Vulnerable Code:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
Issue:
- Unauthorized Access: Users can access data they shouldn't by modifying the orderId parameter.
Mitigation:
Validate resource ownership before providing access.
Improved Code:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Ownership Verification: Ensures that the requested resource belongs to the authenticated user.
- Access Control: Prevents users from accessing others' data by manipulating request parameters.
Session Management Testing (WSTG-SESS)
Session management is critical for maintaining user state and ensuring secure interactions.
Tokens Without Expiration Time
Tokens that never expire pose a security risk if they are compromised.
Example Vulnerable Code:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Issue:
- Persistent Tokens: Tokens without expiration remain valid indefinitely, increasing the window of opportunity for misuse.
Mitigation:
Set an expiration time on tokens.
Improved Code:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Token Expiration: Limits the validity period, reducing the risk if a token is compromised.
- Security Best Practice: Regular token renewal enhances overall security.
Insecure Token Storage
Storing tokens in localStorage exposes them to cross-site scripting (XSS) attacks.
Example Vulnerable Code:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
Issue:
- Client-Side Exposure: Malicious scripts can access localStorage, stealing tokens and hijacking sessions.
Mitigation:
Use HTTP-only cookies to store tokens securely.
Improved Code:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
Explanation:
- HTTP-only Cookies: Inaccessible to JavaScript, mitigating XSS risks.
- Secure and SameSite Flags: Enhance protection against man-in-the-middle and cross-site request forgery attacks.
Input Validation Testing (WSTG-INPV)
Input validation ensures that user-provided data is safe and expected, preventing injection attacks.
Lack of Input Validation
Accepting and processing user input without validation can lead to vulnerabilities.
Example Vulnerable Code:
const express = require('express'); const jwt = require('jsonwebtoken'); const app = express(); // Weak secret key const SECRET_KEY = 'secret'; app.post('/login', (req, res) => { // Authenticate user (authentication logic not shown) const userId = req.body.userId; // Sign the JWT with a weak secret const token = jwt.sign({ userId }, SECRET_KEY); res.json({ token }); }); app.get('/protected', (req, res) => { const token = req.headers['authorization']; try { // Verify the token using the weak secret const decoded = jwt.verify(token, SECRET_KEY); res.send('Access granted to protected data'); } catch (err) { res.status(401).send('Unauthorized'); } }); app.listen(3000, () => { console.log('Server started on port 3000'); });
Issue:
- Injection Attacks: Unvalidated input can lead to SQL injection, NoSQL injection, or other code injection attacks.
Mitigation:
Validate and sanitize all user inputs.
Improved Code:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Input Validation: Checks that input meets expected criteria.
- Input Sanitization: Removes or escapes potentially harmful characters.
- Secure Database Queries: Using parameterized queries prevents injection attacks.
Testing for Error Handling (WSTG-ERRH)
Proper error handling avoids disclosing sensitive information and improves user experience.
Exposing Sensitive Error Information
Detailed error messages can reveal system internals to attackers.
Example Vulnerable Code:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Issue:
- Information Disclosure: Attackers can gain insights into your application's structure and potential vulnerabilities.
Mitigation:
Use generic error messages and log detailed errors internally.
Improved Code:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Internal Logging: Keeps detailed error information secure.
- User-Friendly Messages: Provides a generic message without revealing sensitive details.
Testing for Weak Cryptography (WSTG-CRYP)
Cryptography protects sensitive data; using weak cryptographic practices undermines security.
Using Insecure Hashing Algorithms
Hashing passwords with outdated algorithms is insecure.
Example Vulnerable Code:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
Issue:
- Weak Hashing: Algorithms like MD5 and SHA-1 are vulnerable to collision attacks and should not be used for password hashing.
Mitigation:
Use a strong hashing algorithm designed for passwords.
Improved Code:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
Explanation:
- Bcrypt: A robust hashing function that incorporates salting and multiple rounds of hashing.
- Password Security: Makes it computationally infeasible for attackers to reverse-engineer passwords.
Hardcoding Secret Keys
Storing secrets directly in code increases the risk of exposure.
Example Vulnerable Code:
const express = require('express'); const jwt = require('jsonwebtoken'); const app = express(); // Weak secret key const SECRET_KEY = 'secret'; app.post('/login', (req, res) => { // Authenticate user (authentication logic not shown) const userId = req.body.userId; // Sign the JWT with a weak secret const token = jwt.sign({ userId }, SECRET_KEY); res.json({ token }); }); app.get('/protected', (req, res) => { const token = req.headers['authorization']; try { // Verify the token using the weak secret const decoded = jwt.verify(token, SECRET_KEY); res.send('Access granted to protected data'); } catch (err) { res.status(401).send('Unauthorized'); } }); app.listen(3000, () => { console.log('Server started on port 3000'); });
Issue:
- Secret Exposure: If the codebase is compromised, hardcoded secrets can be easily extracted.
Mitigation:
Store secrets in environment variables or secure configuration files.
Improved Code:
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Environment Variables: Keep secrets out of the codebase and version control systems.
- Security Practices: Reduces the risk of accidental exposure.
Business Logic Testing (WSTG-BUSL)
Business logic vulnerabilities occur when application flows can be manipulated in unintended ways.
Abuse of Bulk Operations
Unrestricted data operations can lead to performance issues or data leakage.
Example Vulnerable Code:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Issue:
- Denial of Service (DoS): Large data exports can exhaust server resources.
- Data Leakage: Unrestricted access may expose sensitive information.
Mitigation:
Implement pagination and access controls.
Improved Code:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Pagination: Controls the amount of data returned, preventing resource exhaustion.
- Access Control: Ensures users can only access their own data.
Client-side Testing (WSTG-CLNT)
Protecting against client-side vulnerabilities is essential to safeguard users from attacks such as Cross-Site Scripting (XSS).
Escaping User Input Using the xss Library
Improper handling of user input in client-side scripts can lead to XSS attacks.
Example Vulnerable Code:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
Issue:
- Unsafe DOM Manipulation: Inserting unsanitized user input into innerHTML allows execution of malicious scripts.
Mitigation:
Use the xss library to sanitize user input before rendering.
Improved Code:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
Explanation:
- Input Sanitization: The xss library cleans input by escaping or removing potentially dangerous content.
- Preventing Script Execution: Neutralizes malicious scripts, preventing them from executing in the browser.
Best Practices:
- Use textContent When Possible: Assigning user input to textContent treats it as plain text.
const express = require('express'); const app = express(); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
- Combine Client and Server-side Validation: A defense-in-depth approach enhances security.
API Testing (WSTG-APIT)
Securing API endpoints is crucial to prevent data leaks and unauthorized access.
GraphQL Introspection Exposure
Leaving GraphQL introspection enabled in production reveals your API schema.
Example Vulnerable Code:
const express = require('express'); const app = express(); // Disable the X-Powered-By header app.disable('x-powered-by'); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Issue:
- Schema Disclosure: Attackers can explore your API schema, aiding in crafting targeted attacks.
Mitigation:
Disable introspection in production environments.
Improved Code:
const express = require('express'); const helmet = require('helmet'); const app = express(); // Use Helmet to secure headers app.use(helmet()); // Your routes here app.listen(3000, () => { console.log('Server is running on port 3000'); });
Explanation:
- Conditional Introspection: Allows introspection during development but disables it in production.
- Security Enhancement: Reduces the attack surface by hiding schema details.
Unrestricted Query Complexity
Deeply nested or complex queries can exhaust server resources.
Example Vulnerable Code:
// app.js const express = require('express'); const app = express(); // Error handling middleware app.use((err, req, res, next) => { res.status(500).send(err.stack); // Sends stack trace to the client }); // Your routes here app.listen(3000);
Issue:
- Denial of Service (DoS): Complex queries can lead to high CPU and memory usage.
Mitigation:
Limit query depth and complexity.
Improved Code:
// app.js const express = require('express'); const app = express(); // Your routes here // Error handling middleware if (app.get('env') === 'production') { // Production error handler app.use((err, req, res, next) => { // Log the error internally console.error(err); res.status(500).send('An unexpected error occurred.'); }); } else { // Development error handler (with stack trace) app.use((err, req, res, next) => { res.status(500).send(`<pre class="brush:php;toolbar:false">${err.stack}`); }); } app.listen(3000);
Explanation:
- Depth Limiting: Restricts the depth of queries to prevent resource exhaustion.
- Performance Protection: Ensures the API remains responsive and available.
Conclusion
Securing your Node.js application involves a multi-layered approach:
- Prevent Information Leakage: Clean up code and server configurations to avoid exposing sensitive data.
- Manage Configurations Securely: Remove default credentials and secure configuration files.
- Validate and Sanitize Input: Never trust user input.
- Implement Proper Authentication and Authorization: Ensure users have appropriate access.
- Use Strong Cryptography: Protect data with secure algorithms and key management.
- Handle Errors Gracefully: Avoid revealing sensitive information.
- Protect Client-side Interactions: Mitigate XSS and other browser-based attacks.
- Secure APIs: Control data exposure and enforce rate limiting.
By integrating these practices, you enhance your application's security, protect user data, and maintain trust.
Further Reading
- OWASP Web Security Testing Guide (WSTG): OWASP WSTG
- Node.js Security Guide: Node.js Security
- Express.js Security Tips: Express Security Best Practices
- GraphQL Security Best Practices: Apollo GraphQL Security
- OWASP Top Ten: OWASP Top Ten
- MDN Web Docs - Web Security: MDN Web Security
Note: This guide provides general recommendations. For specific security concerns, consult a professional.
The above is the detailed content of Securing Your Node.js Application: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




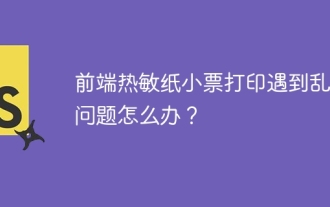
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
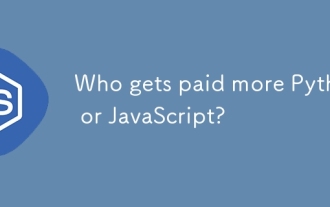
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
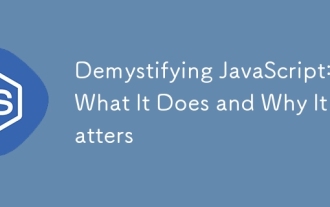
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
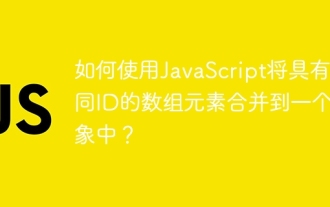
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
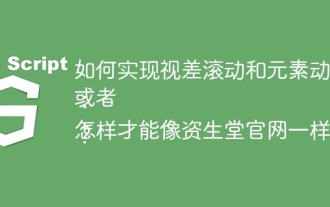
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
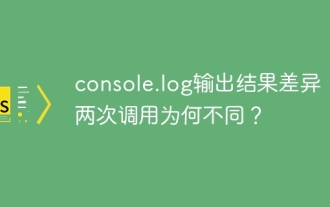
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
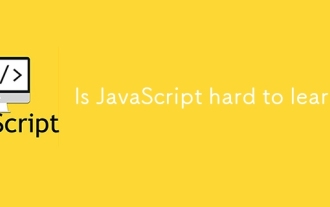
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
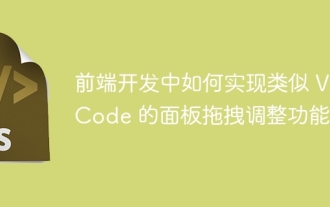
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
