How Can I Simulate Python's Zip Function Using C 11 and Beyond?
Zipping Sequences in C 11
With the introduction of range-based for-loops in C 11, code has become more concise and readable. However, the question arises whether it can be used to simulate Python's zip function, which iterates over multiple collections simultaneously.
Boost Zip Functionality
Although the range-based for-loop does not directly support simultaneous loops, the Boost library provides a zip_iterator. It allows users to define a range that iterates over elements from multiple containers in parallel.
Usage of Boost Zip_iterator
The following code snippet demonstrates how to use boost::zip_iterator:
#include <boost/iterator/zip_iterator.hpp> #include <vector> int main() { std::vector<int> a {1, 2, 3}; std::vector<std::string> b {"one", "two", "three"}; for (auto tup : boost::make_zip_iterator(boost::make_tuple(a.begin(), b.begin()))) { int x; std::string y; boost::tie(x, y) = tup; std::cout << x << " " << y << std::endl; } }
This code will output:
1 one 2 two 3 three
Warning:
It is important to note that the length of all input containers must be equal. Otherwise, undefined behavior may occur.
Alternatives
Besides Boost, there are alternative libraries that offer zip functionality for C . For example, the Ranges library provides a zip_view that can be used with range-based for-loops:
#include <ranges> int main() { std::vector<int> a {1, 2, 3}; std::vector<std::string> b {"one", "two", "three"}; for (auto [x, y] : std::ranges::zip_view(a, b)) { std::cout << x << " " << y << std::endl; } }
Whether to use Boost or other libraries depends on the specific requirements and project setup.
The above is the detailed content of How Can I Simulate Python's Zip Function Using C 11 and Beyond?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










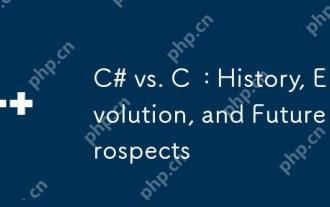
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
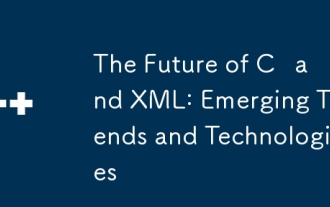
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
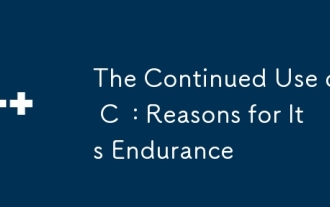
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
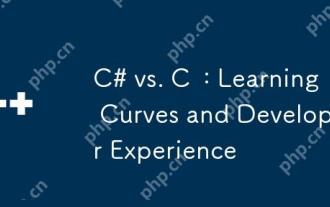
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
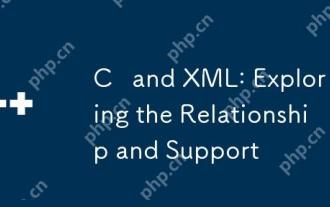
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
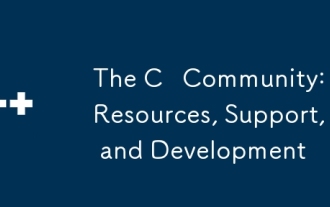
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
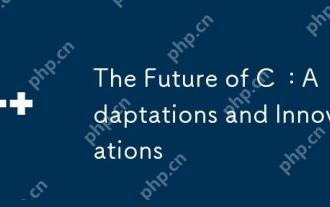
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
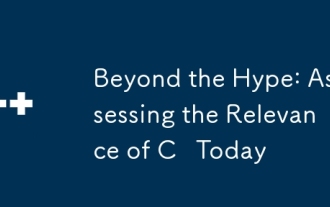
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
