


How to Customize the Printing of Go Structs Without Explicitly Defining a String() Method for Each Struct?
Printing Structs with Custom Field Formatting
While fmt.Println conveniently displays built-in types, its behavior for structs can be verbose and uninformative. Consider a struct representing a time value with additional fields:
type A struct { t time.Time }
Printing this struct using fmt.Println results in:
{{63393490800 0 0x206da0}}
which is not readily interpretable. Specifically, the struct lacks a String() method, which prevents its fields from being formatted as desired.
Problem:
How can we print a struct with custom string representations for its fields, without explicitly defining a String() method for each struct?
Solution:
Reflection can be used to iterate over the fields of a struct and call their String() methods dynamically. Here's a helper function that accomplishes this:
func PrintStruct(s interface{}, names bool) string { v := reflect.ValueOf(s) t := v.Type() // Handle non-struct input if t.Kind() != reflect.Struct { return fmt.Sprint(s) } // Initialize buffer b := &bytes.Buffer{} b.WriteString("{") for i := 0; i < v.NumField(); i++ { if i > 0 { b.WriteString(" ") } v2 := v.Field(i) if names { b.WriteString(t.Field(i).Name) b.WriteString(":") } // Handle Stringer fields if v2.CanInterface() { if st, ok := v2.Interface().(fmt.Stringer); ok { b.WriteString(st.String()) continue } } // Print non-Stringer fields fmt.Fprint(b, v2) } b.WriteString("}") // Return formatted string return b.String() }
Usage:
This function can be used to print a struct with custom field formatting:
a := A{time.Now()} fmt.Println(PrintStruct(a, true)) // Display field names fmt.Println(PrintStruct(a, false)) // Omit field names
Notes:
- The PrintStruct function assumes exported struct fields.
-
For added convenience, you can define a String() method for your struct that simply calls PrintStruct:
func (a A) String() string { return PrintStruct(a, true) }
Copy after login
The above is the detailed content of How to Customize the Printing of Go Structs Without Explicitly Defining a String() Method for Each Struct?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










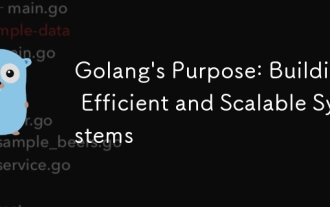
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
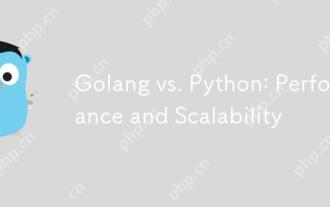
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
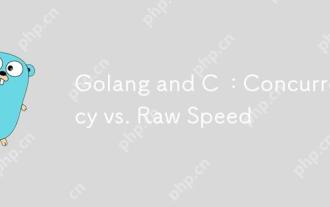
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
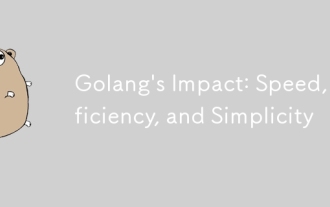
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
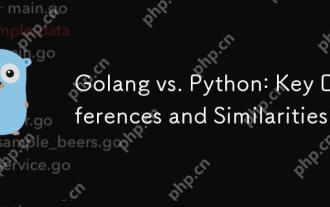
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
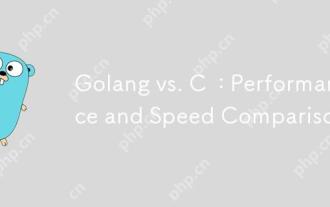
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
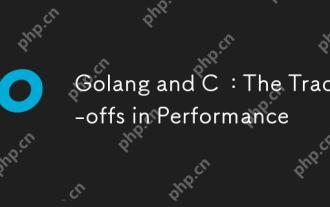
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
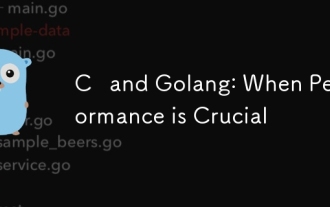
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
