


How to Debug and Correctly Execute Commands in Kubernetes Pods Using the Go Client?
Kubernetes Pod Execution Using Go Client
You want to execute a command inside a pod using the Kubernetes Go client, but your current implementation is encountering an error in exec.Stream(sopt) without any error message. This article will guide you through debugging and provides a correct example for your use case.
Debugging the Issue
The current error could arise due to incorrect configuration parameters or mismatched versions. Verify the following:
- Ensure that the Host field in your config matches the Kubernetes API server address.
- Update config.ContentConfig.GroupVersion to use the correct Kubernetes API version supported by your cluster.
- Check if the container name provided in req.VersionedParams matches the name of an existing container within the specified pod.
Correct Implementation
Here's a corrected example based on the modified ExecCmdExample function:
package k8s import ( "io" v1 "k8s.io/api/core/v1" "k8s.io/client-go/kubernetes" _ "k8s.io/client-go/plugin/pkg/client/auth/gcp" // Auth plugin specific to GKE "k8s.io/client-go/rest" "k8s.io/client-go/tools/remotecommand" ) // ExecCmdExample executes a command on a specific pod and waits for the command's output. func ExecCmdExample(client kubernetes.Interface, podName string, command string, stdin io.Reader, stdout io.Writer, stderr io.Writer) error { // Use a larger reader buffer size to handle long outputs. buf := make([]byte, 10000) cmd := []string{ "sh", "-c", command, } options := &v1.PodExecOptions{ Command: cmd, Stdin: stdin != nil, Stdout: true, Stderr: true, TTY: false, } req := client.CoreV1().RESTClient().Post(). Resource("pods"). Name(podName). Namespace("default"). SubResource("exec"). VersionedParams( options, scheme.ParameterCodec, ) exec, err := remotecommand.NewSPDYExecutor(config, "POST", req.URL()) if err != nil { return err } err = exec.Stream(remotecommand.StreamOptions{ Stdin: stdin, Stdout: stdout, Stderr: stderr, }) // Read additional output if necessary. if _, err = exec.Read(buf); err != nil { return err } return nil }
- The config variable has been removed as it is assumed to be configured elsewhere.
- The VersionedParams method now handles newer versions of PodExecOptions and ParameterCodec.
- A buffered reader is used to handle long command outputs.
- The TTY option is set to false since you don't require interactive terminal support.
The above is the detailed content of How to Debug and Correctly Execute Commands in Kubernetes Pods Using the Go Client?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










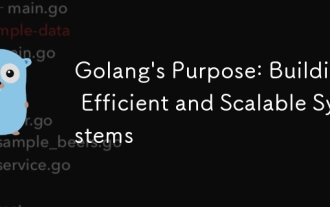
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
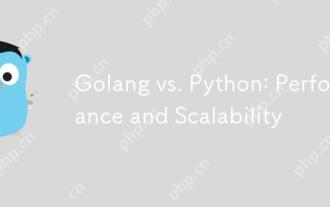
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
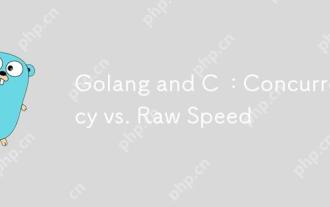
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
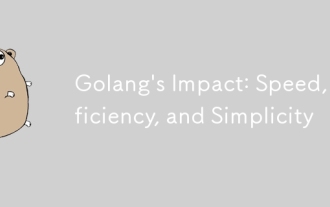
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
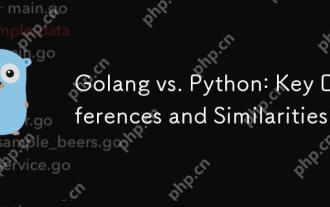
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
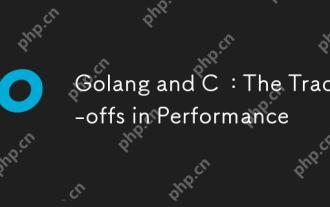
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
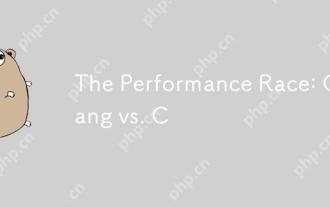
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
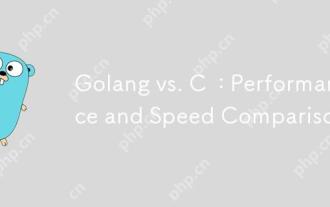
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
