How Can I Extract All Regex Matches into an Array in Java?
Extract String Matches into Array Using Regex Expressions in Java
In Java, the inability to directly obtain an array of regex matches may leave you puzzled. This guide addresses this challenge, providing solutions to capture all strings that conform to a regular expression.
The key to retrieving these matches lies in utilizing a matcher, which iteratively locates occurrences:
import java.util.regex.Matcher; import java.util.regex.Pattern; List<String> allMatches = new ArrayList<>(); Matcher m = Pattern.compile("your regex expression here") .matcher(yourStringHere); while (m.find()) { allMatches.add(m.group()); }
After populating allMatches with the matches, you can convert it to an array if necessary:
allMatches.toArray(new String[0]);
Alternatively, you may consider using MatchResult to simplify matching operations. A helper function like allMatches:
public static Iterable<MatchResult> allMatches( final Pattern p, final CharSequence input) { ... }
allows you to iterate over matches, such as:
for (MatchResult match : allMatches(Pattern.compile("[abc]"), "abracadabra")) { System.out.println(match.group() + " at " + match.start()); }
Providing the output:
a at 0 b at 1 a at 3 c at 4 a at 5 a at 7 b at 8 a at 10
The above is the detailed content of How Can I Extract All Regex Matches into an Array in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










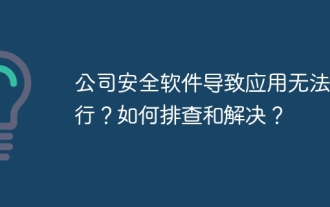
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
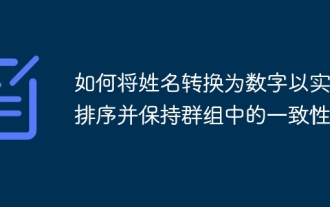
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
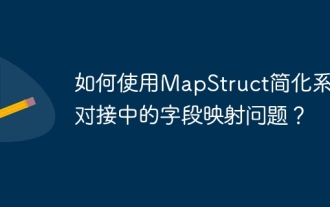
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
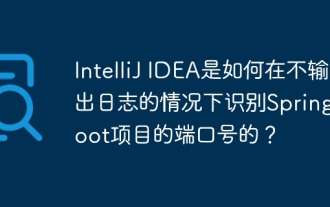
Start Spring using IntelliJIDEAUltimate version...
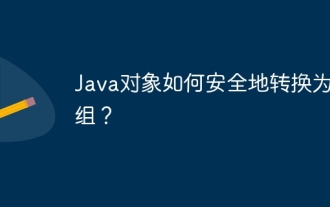
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
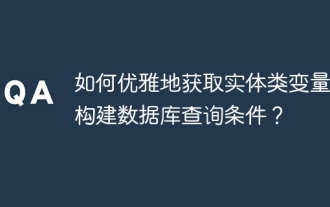
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
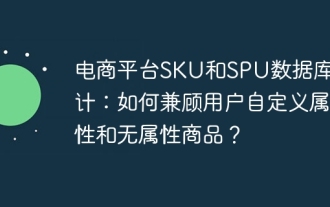
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
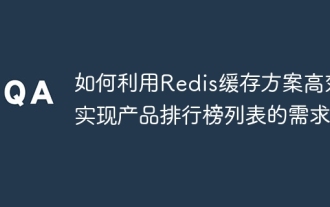
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
