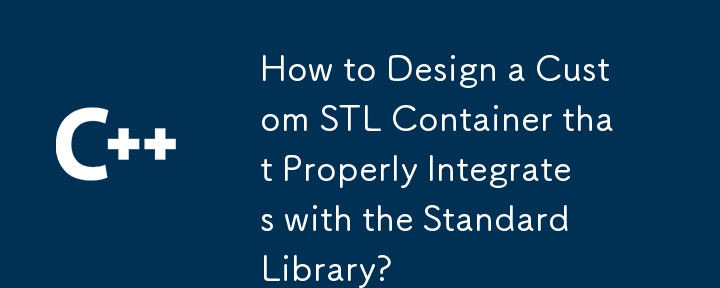
The Guidelines for Writing a Custom STL Container
When designing a new container that adheres to the STL conventions, it's crucial to follow certain guidelines to ensure its proper behavior and integration with the STL library.
Iterator Interface:
- Define an iterator class with an appropriate iterator_category tag, such as input_iterator_tag, output_iterator_tag, forward_iterator_tag, bidirectional_iterator_tag, or random_access_iterator_tag.
- Provide member functions for comparison (==, !=), increment/decrement ( , --), arithmetic ( , -), and dereferencing (*, ->) operations.
Const Iterator:
- Provide a const_iterator class nested within the iterator class, allowing for iteration over constant containers.
- Define the same member functions as the non-const iterator, but with const references and pointers.
Comparison and Assignment:
- Implement comparison operators (==, !=, <, >, <=, >=) for the container itself.
- Provide an assignment operator that takes a reference to another container.
Capacity and Access:
- Include member functions such as empty(), size(), max_size() to manage container size and capacity.
- Provide begin(), end(), cbegin(), cend(), rbegin(), rend(), crbegin(), and crend() for accessing iterators representing the container's elements.
- Define functions like front(), back(), at(), and operator[] to access or modify elements.
Insertions and Deletions:
- Implement emplace_front(), emplace_back(), push_front(), push_back(), pop_front(), and pop_back() for element insertion and deletion.
- Provide emplace(), insert(), and erase() member functions for inserting and removing elements within the container.
Miscellaneous:
- Include a swap() member function to exchange the contents of two containers of the same type.
- Implement get_allocator() to retrieve the allocator associated with the container.
- Define a free-standing swap() function for swapping containers of the same type.
Testing:
To ensure the reliability of your container, use a testing class like the provided tester class to verify that:
- Object lifetime is properly managed.
- Function calls do not modify the object's state unexpectedly.
- The container functions correctly when created with and without global objects.
The above is the detailed content of How to Design a Custom STL Container that Properly Integrates with the Standard Library?. For more information, please follow other related articles on the PHP Chinese website!