Why Does My Random Number Generator Keep Producing the Same Sequence?
Random Number Generator Resetting to the Same Sequence
Each time you execute a program that utilizes the rand() function, you might notice it produces identical sequences of numbers. This occurs because the random number generator's seed is not initialized.
To produce more unpredictable results, set the random number generator's seed using srand((unsigned int)time(NULL)). This function employs the current time as the seed, providing more variety in the generated numbers. Consider the following code:
#include <iostream> #include <cstdlib> #include <ctime> using namespace std; int random(int low, int high) { if (low > high) return high; return low + (rand() % (high - low + 1)); } int main() { srand((unsigned int)time(NULL)); cout << random(2, 5) << endl; return 0; }
The rand() function is not inherently random, but rather utilizes a mathematical transformation. Each call to rand() generates a result based on the seed or previous random numbers, creating a predictable sequence without explicit initialization. By using srand with a truly random value (like the current time), the random number generator's state becomes more unpredictable, leading to more varied results.
For further insights, refer to these resources:
- http://www.dreamincode.net/forums/topic/24225-random-number-generation-102/
- http://www.dreamincode.net/forums/topic/29294-making-pseudo-random-number-generators-more-random/
The above is the detailed content of Why Does My Random Number Generator Keep Producing the Same Sequence?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


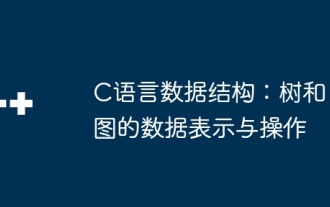
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
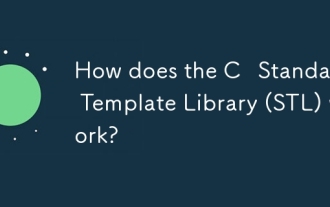
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
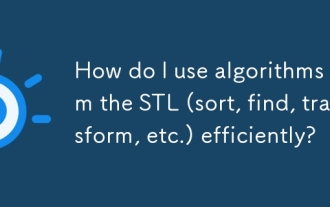
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
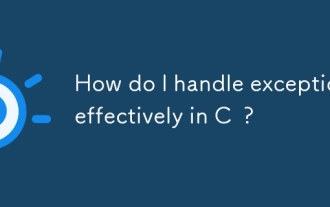
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
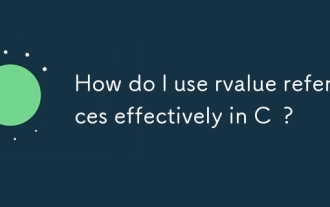
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
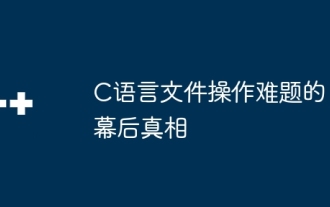
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
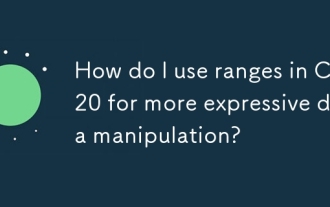
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
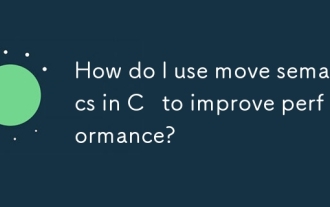
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
