How to Efficiently Iterate Through a JSON Array in Android/Java?
Dec 09, 2024 am 02:50 AMJSON Array Iteration in Android/Java
Accessing a JSON object from an Android app requires decoding the JSON array. To iterate through the array and extract the desired data, several approaches can be considered.
Looping through JSONArray
A straightforward method is to loop through the JSONArray using a for loop:
int id; String name; JSONArray array = new JSONArray(jsonString); for (int i = 0; i < array.length(); i++) { JSONObject row = array.getJSONObject(i); id = row.getInt("id"); name = row.getString("name"); }
Using a Library
Alternatively, utilizing a third-party library like Gson or Jackson simplifies the JSON parsing process. For instance, using Gson:
Gson gson = new Gson(); Type type = new TypeToken<List<JsonObject>>() {}.getType(); List<JsonObject> rows = gson.fromJson(jsonString, type);
Iterable Approach
Another option is to convert the JSONArray to an iterable using the JSONPointer class:
JSONArray array = new JSONArray(jsonString); JSONPointer pointer = new JSONPointer("/0"); int id = (int) pointer.getValue(array); String name = (String) pointer.getValue(array, "/1");
Legacy Solution
The code snippet mentioned in the question required an implicit conversion from the JSONArray to an iterable array. Unfortunately, this approach may encounter compatibility issues and is not recommended.
Optimization
For large datasets, consider using asynchronous tasks or background threads to avoid blocking the main UI. Additionally, caching the parsed data can enhance performance.
The above is the detailed content of How to Efficiently Iterate Through a JSON Array in Android/Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
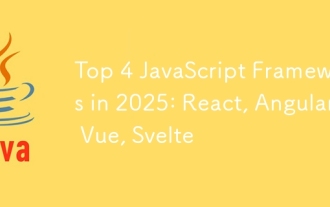
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
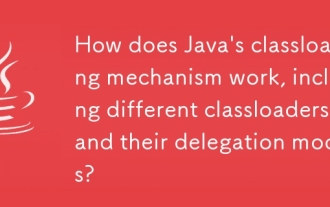
How does Java's classloading mechanism work, including different classloaders and their delegation models?
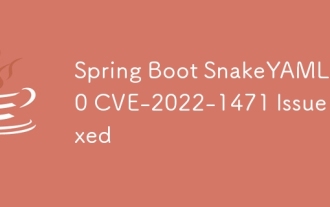
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
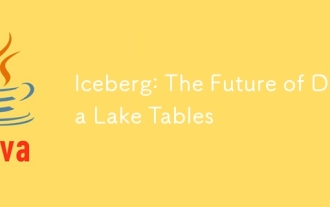
Iceberg: The Future of Data Lake Tables
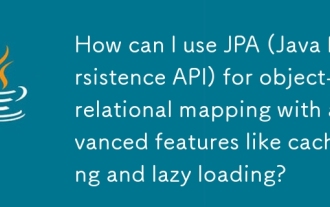
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
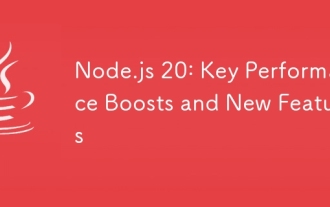
Node.js 20: Key Performance Boosts and New Features
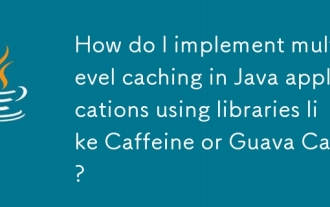
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
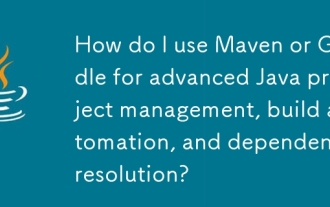
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
